#include <gcroot.h>
时间: 2024-06-12 17:02:46 浏览: 135
`<gcroot.h>`是Microsoft的垃圾回收(Garbage Collection, GC)框架的一部分,在.NET平台上,尤其是C#中使用,用于与垃圾回收器进行交互。这个头文件通常在需要直接操作底层的GC数据结构或执行低级别的内存管理操作时使用。
当你包含`<gcroot.h>`,你获得了访问`gcroot`类型的能力,这是一种特殊的指针,它可以安全地指向托管堆中的对象,即使这些对象已经超出其作用域并且会被垃圾回收器清理。`gcroot`提供了跨线程和生命周期管理的安全途径,允许你在不受GC控制的情况下保持对对象的引用。
使用`gcroot`需要注意的是,这通常用于性能敏感或者特定情况下需要对GC内部细节进行直接控制的场景,比如写入底层库、互斥锁或其他同步结构时。
相关问题
#include <stdio.h> #include <stdlib.h> #include <X11/Xlib.h> #include <X11/Xutil.h> #include <libEMF/emf.h> int main(int argc, char **argv) { EMF_HANDLE handle; EMR *record; int i; if (argc < 2) { printf("Usage: %s <filename.emf>\n", argv[0]); return 1; } handle = EMF_LoadFile(argv[1]); if (handle == NULL) { printf("Failed to open file %s\n", argv[1]); return 2; } Display *display = XOpenDisplay(NULL); if (!display) { printf("Failed to open X11 display\n"); return 3; } int screen = DefaultScreen(display); Window root_window = RootWindow(display, screen); Window window = XCreateSimpleWindow(display, root_window, 0, 0, 640, 480, 0, BlackPixel(display, screen), WhitePixel(display, screen)); XSelectInput(display, window, ExposureMask | KeyPressMask); XMapWindow(display, window); GC gc = XCreateGC(display, window, 0, NULL); while (1) { XEvent event; XNextEvent(display, &event); if (event.type == Expose) { for (i = 0; i < EMF_GetNumRecords(handle); i++) { record = EMF_GetRecord(handle, i); // 处理EMF记录,绘制到窗口中 switch (record->iType) { case EMR_HEADER: // 处理EMF文件头 break; case EMR_POLYLINE: { EMRPOLYLINE *polyline = (EMRPOLYLINE *)record; XPoint *points = malloc(polyline->cptl * sizeof(XPoint)); int j; for (j = 0; j < polyline->cptl; j++) { points[j].x = polyline->aptl[j].x; points[j].y = -polyline->aptl[j].y; // Y坐标需要翻转 } XDrawLines(display, window, gc, points, polyline->cptl, CoordModeOrigin); free(points); break; } // 处理其他EMF记录类型 default: break; } } } else if (event.type == KeyPress) { break; } } XFreeGC(display, gc); XDestroyWindow(display, window); XCloseDisplay(display); EMF_CloseFile(handle); return 0; }
这段代码使用libEMF库和Xlib库来展示emf文件的内容。
首先,我们使用`EMF_LoadFile()`函数加载emf文件,并创建一个X窗口。然后,我们进入一个事件循环,等待窗口曝光事件或按键事件的发生。当窗口曝光事件发生时,我们遍历emf文件中的所有记录,处理每个记录并将其绘制到窗口中。具体来说,我们使用Xlib库中的`XDrawLines()`函数来绘制线段。
需要注意的是,由于X窗口的坐标系与emf文件的坐标系不同,我们需要将emf文件中的Y坐标进行翻转。
以下是示例代码:
用C语言编写程序,利用拓展的后序遍历创建二叉树,拓展的后序遍历序列为AB.DF..GC.E.H..,.表示空树
以下是用C语言编写的程序,利用拓展的后序遍历创建二叉树,拓展的后序遍历序列为AB.DF..GC.E.H..,.表示空树的代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义二叉树节点的结构体
typedef struct TreeNode {
char val; // 节点的值
struct TreeNode *left; // 左子树指针
struct TreeNode *right; // 右子树指针
} TreeNode;
// 递归创建二叉树的函数
TreeNode* createTree(char *postorder, int *index) {
// 取出当前节点的值
char val = postorder[(*index)--];
// 如果当前节点为空树,返回空节点
if (val == '.') {
return NULL;
}
// 创建一个新节点
TreeNode *node = (TreeNode*) malloc(sizeof(TreeNode));
node->val = val;
// 递归构建右子树
node->right = createTree(postorder, index);
// 递归构建左子树
node->left = createTree(postorder, index);
return node;
}
int main() {
// 定义拓展的后序遍历序列
char postorder[] = "AB.DF..GC.E.H..";
// 定义节点索引
int index = strlen(postorder) - 1;
// 创建二叉树
TreeNode *root = createTree(postorder, &index);
return 0;
}
```
该程序首先定义了二叉树节点的结构体,然后定义了一个递归创建二叉树的函数createTree。该函数的参数包括拓展的后序遍历序列和节点索引,其中节点索引从末尾开始,每次递归时会自减。函数的执行过程与前面介绍的算法相同。
最后,在main函数中调用createTree函数创建二叉树,并返回0表示程序正常结束。
阅读全文
相关推荐
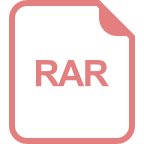
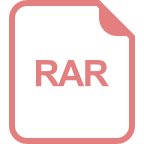
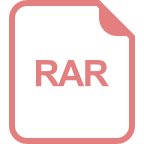
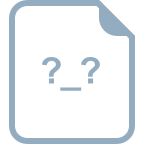
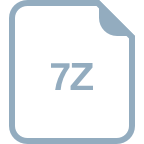
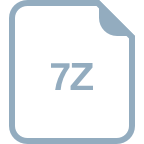
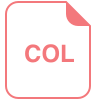
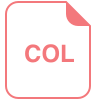
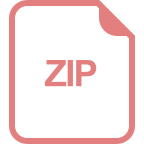
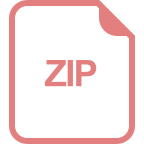