创建一个类,其中包括一个转换成N进制和一个转换成十进制,编写转换和输出方法
时间: 2024-09-29 13:08:29 浏览: 17
当你想创建一个类来处理数字的进制转换时,你可以定义一个`NumberConverter`类,其中包含两个方法:一个是将十进制转换为任意指定的进制(比如二进制、八进制或十六进制),另一个是将其他进制的数转换回十进制。这里是一个简单的示例:
```java
public class NumberConverter {
// 将十进制转为任意进制的方法
public String decimalToBase(int decimal, int base) {
if (base < 2 || base > 36) {
throw new IllegalArgumentException("Base must be between 2 and 36");
}
StringBuilder result = new StringBuilder();
while (decimal != 0) {
int remainder = decimal % base;
if (remainder < 10) {
result.append(remainder);
} else {
result.append((char) ('A' + remainder - 10));
}
decimal /= base;
}
return result.reverse().toString(); // 由于数学计算是从低位到高位,结果需要反转
}
// 将其他进制的字符串转换回十进制
public int baseToDecimal(String input, int base) {
if (base < 2 || base > 36) {
throw new IllegalArgumentException("Base must be between 2 and 36");
}
int decimal = 0;
for (int i = 0; i < input.length(); i++) {
char digit = input.charAt(i);
if (Character.isDigit(digit)) {
decimal = decimal * base + digit - '0';
} else {
decimal = decimal * base + digit - 'A' + 10;
}
}
return decimal;
}
// 输出方法,用于演示如何使用这两个方法
public void convertAndOutput(String input, int fromBase, int toBase) {
int decimalInput = baseToDecimal(input, fromBase);
String convertedToBase = decimalToBase(decimalInput, toBase);
System.out.printf("Input (%s in Base %d) is %s in Base %d.\n", input, fromBase, convertedToBase, toBase);
}
}
// 使用示例
public static void main(String[] args) {
NumberConverter converter = new NumberConverter();
converter.convertAndOutput("15", 10, 2); // 十进制15转换为二进制
}
```
这个类提供了基本的进制转换功能。要使用它,只需实例化该类并调用`convertAndOutput`方法,传入你想要转换的数值、原始基数和目标基数。
相关推荐
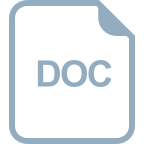
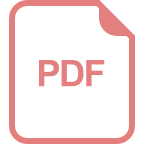

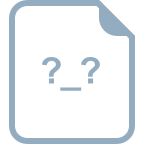
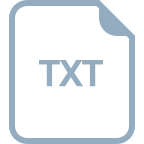
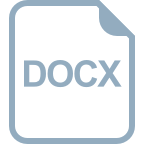
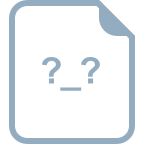
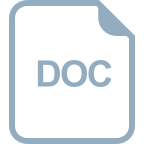








