void swap(int p1,int p2) //定义swap函数 { int temp; }
时间: 2023-12-13 11:03:22 浏览: 20
你定义的`swap`函数中缺少了实现交换两个变量值的代码。在你提供的代码中,`temp`变量没有被使用,也没有将`p1`和`p2`的值进行交换。
下面是一个完整的交换两个变量值的示例代码:
```c
void swap(int* p1, int* p2) {
int temp = *p1;
*p1 = *p2;
*p2 = temp;
}
```
在这个示例中,我们使用指针作为函数参数,通过指针来操作变量的值。`p1`和`p2`是指向整型变量的指针。我们使用一个临时变量`temp`来保存`p1`指向的值,然后将`p2`指向的值赋给`p1`,最后将`temp`的值赋给`p2`,实现了两个变量值的交换。
希望这能帮助到你。如果还有其他问题,请随时提问。
相关问题
void swap(int *p1,int *p2) //定义swap函数 { int *temp; *temp=*p1; *p1=*p2; *p2=*temp; } 为什么这个不对
你提供的代码有一个问题,即`temp`指针没有被正确初始化。在执行`*temp = *p1;`这行代码时,由于`temp`指针没有指向任何有效的内存位置,会导致未定义的行为。
为了修正这个问题,你需要为`temp`指针分配内存空间,或者使用一个已经存在的变量来作为中间变量。下面是两种修正的方法:
1. 使用已经存在的变量作为中间变量:
```c
void swap(int *p1, int *p2) {
int temp = *p1;
*p1 = *p2;
*p2 = temp;
}
```
2. 分配内存空间给`temp`指针:
```c
void swap(int *p1, int *p2) {
int *temp = (int*)malloc(sizeof(int));
*temp = *p1;
*p1 = *p2;
*p2 = *temp;
free(temp); // 释放内存空间
}
```
这两种修正方法都可以正确地交换两个指针所指向的值。
希望能够帮助到你。如果还有其他问题,请随时提问。
void swap( int* p1,int* p2 )
### 回答1:
您好!以下是用中文回答您的问题:
这是一个交换两个整数变量值的函数,参数分别是两个整型指针。
函数代码如下:
void swap(int* p1, int* p2) {
int temp = *p1;
*p1 = *p2;
*p2 = temp;
}
函数使用指针作为参数,因为它需要修改变量的值。在函数内部,它将变量p1所指向的值存储在临时变量temp中,然后将p2所指向的值复制给p1所指向的值,最后将temp的值复制给p2所指向的值,从而完成了两个变量值的交换。
希望这能够帮到您!
### 回答2:
函数声明中的void swap( int* p1,int* p2 )表示定义了一个名为swap的函数,它需要两个int类型的指针p1和p2作为参数。这意味着,在使用这个swap函数之前,我们需要用正确的参数来调用它,这样才能正确地执行交换操作。
当我们在swap函数中使用这两个指针时,它实际上会交换它们所指向的值。对于指针p1和p2,swap函数首先通过使用临时变量将指针p1指向的值存储在临时变量temp中。然后,它将指针p2指向的值复制到指针p1指向的地址,即将p2指向的值赋值给p1指向的位置。最后,函数将在temp中存储的原始值复制到p2指向的地址,即将temp中的值赋值给p2指向的位置。
使用该函数可以轻松地实现对两个变量的交换操作,这对于排序和其他算法实现非常有用。我们可以将该函数用于任何需要交换两个变量的地方,只需将变量的地址传递给函数即可。
### 回答3:
void swap(int* p1, int* p2)是一个定义在C语言和C++中的函数,其功能是交换指针p1和p2所指向的两个整型变量的值。
首先,我们需要了解指针是什么。指针是一个变量,其存储的是内存地址。在C/C++中,我们可以用指针来访问内存中的数据,也可以通过指针来修改内存中的数据。
在swap函数中,p1和p2是两个指向整型变量的指针。通过*p1和*p2,我们可以访问和修改p1和p2所指向的变量的值。
在函数内部,我们首先定义一个中间变量temp来存储p1所指向的变量的值,然后将p1所指向的变量的值改为p2所指向的变量的值,最后将p2所指向的变量的值改为temp,即完成了两个变量的值的交换。
其中,符号*用于取出指针指向的地址中的值,指针变量与普通变量的区别在于它存储的是地址而不是值,因此要用*来获取它所指向的值;&符号用于获取变量的地址。
需要注意的是,调用swap函数时,传入的必须是两个整型变量的地址,而不能是变量本身。因此,我们使用&运算符来获取变量的地址,如下所示:
int a = 1, b = 2;
swap(&a, &b);
这样就可以将变量a和b的值交换了。
总之,void swap(int* p1, int* p2)是一个非常常用的函数。通过指针,我们可以在函数内部修改外部变量的值,从而实现各种功能。
相关推荐
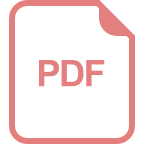












