用c语言,编一个程序把十进制数转为二进制
时间: 2024-10-10 13:14:15 浏览: 25
在 C 语言中,你可以通过位运算来将一个十进制整数转换成二进制。下面是一个简单的程序示例:
```c
#include <stdio.h>
#include <math.h>
// Function to convert decimal to binary
void dec_to_bin(int num) {
int binary[32]; // Maximum length for a 32-bit integer in binary is 31 bits
int i = 0;
while (num > 0) {
binary[i] = num % 2; // Get the remainder (0 or 1)
num /= 2; // Divide by 2 and shift left
i++;
}
// Print the binary representation from right to left
for (int j = i - 1; j >= 0; j--) {
printf("%d", binary[j]);
}
}
int main() {
int decimal;
printf("Enter a decimal number: ");
scanf("%d", &decimal);
printf("Binary representation of %d is ", decimal);
dec_to_bin(decimal);
printf("\n");
return 0;
}
```
这个程序首先定义了一个函数 `dec_to_bin`,它接收一个十进制数字,然后用循环将其逐步除以2并保存余数(即二进制的每一位),最后从低位到高位打印出来。注意,这里假设输入的数字不超过32位(对于大多数现代计算机而言,这是合理的假设)。
运行此程序时,输入一个十进制数值,它会输出对应的二进制表示。如果你想要获取更多关于如何编写此类程序的信息,可以问我相关的
相关推荐
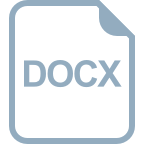
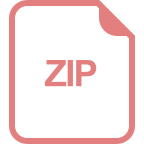














