python图像翻转
时间: 2023-08-15 14:14:38 浏览: 136
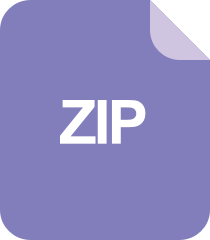
Python PIL图像处理
在Python中,可以使用Pillow库来进行图像处理操作,包括图像翻转。下面是一个简单的示例代码,演示如何实现图像的水平翻转和垂直翻转:
```python
from PIL import Image
# 打开图像文件
image = Image.open("path/to/image.jpg")
# 水平翻转
flipped_image = image.transpose(Image.FLIP_LEFT_RIGHT)
# 垂直翻转
# flipped_image = image.transpose(Image.FLIP_TOP_BOTTOM)
# 保存翻转后的图像
flipped_image.save("path/to/flipped_image.jpg")
```
在示例代码中,我们使用`Image.transpose()`方法来进行图像的翻转操作。`Image.FLIP_LEFT_RIGHT`表示水平翻转,`Image.FLIP_TOP_BOTTOM`表示垂直翻转。你可以根据需要选择需要的翻转方式,将其作为参数传递给`transpose()`方法即可。
记得将代码中的`"path/to/image.jpg"`替换为你实际的图像文件路径,以及将`"path/to/flipped_image.jpg"`替换为保存翻转后图像的文件路径。
阅读全文
相关推荐
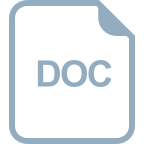
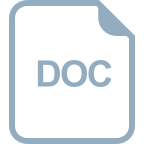


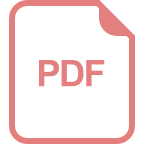
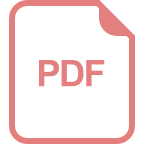
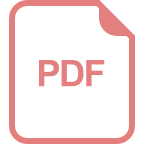
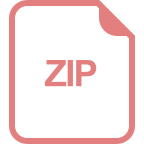









