vue2购物车的商品结算怎么写
时间: 2024-10-13 21:08:45 浏览: 23
在Vue2中实现购物车商品结算功能通常会涉及到组件化设计,以下是一个简单的步骤概述:
1. **创建组件**:
- 创建一个`CartItem.vue`组件,用于显示单个商品的信息,包括名称、价格、数量等,并提供增加和减少数量的操作。
```html
<template>
<div>
<h4>{{ item.name }}</h4>
<p>价格:{{ item.price }}元</p>
<button @click="decreaseQuantity">-</button>
<span>数量:{{ quantity }}</span>
<button @click="increaseQuantity">+</button>
</div>
</template>
<script>
export default {
data() {
return {
quantity: 1,
};
},
methods: {
decreaseQuantity() {
if (this.quantity > 0) {
this.quantity--;
}
},
increaseQuantity() {
this.quantity++;
},
},
};
</script>
```
2. **创建购物车组件**:
- `ShoppingCart.vue`组件将包含一个数组存储所有选中的商品,并处理添加、删除商品以及结算操作。
```html
<template>
<ul>
<li v-for="(item, index) in cartItems" :key="index">
<CartItem :item="item" @updateQuantity="handleQuantityChange"></CartItem>
</li>
<button @click="checkout">结算</button>
</ul>
</template>
<script>
import CartItem from './CartItem.vue';
export default {
components: { CartItem },
data() {
return {
cartItems: [],
};
},
methods: {
addItem(item) {
this.cartItems.push(item);
},
removeItem(item) {
const index = this.cartItems.findIndex((i) => i.id === item.id);
if (index !== -1) {
this.cartItems.splice(index, 1);
}
},
handleQuantityChange(item) {
// 更新cartItems中对应商品的数量
},
checkout() {
// 购物车结算逻辑,计算总价并跳转到订单页面
},
},
computed: {
totalPrice() {
return this.cartItems.reduce((total, item) => total + item.price * item.quantity, 0);
},
},
// 可能需要的事件总线或其他状态管理库来处理数据变化
// ...
};
</script>
```
3. **关联商品数据**:
- 需要在项目中管理商品数据,可以是从API获取、来自JSON文件还是本地存储,根据实际情况决定。
阅读全文
相关推荐
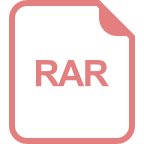
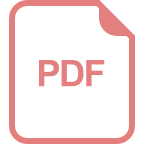
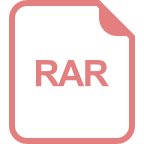
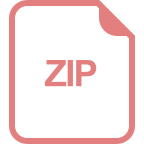
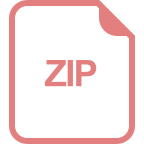
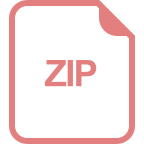
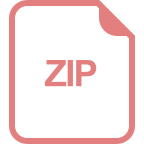
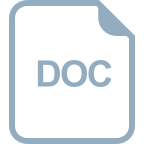






