写出五个简单的c语言程序
时间: 2024-09-11 11:04:48 浏览: 34
当然可以。以下是五个简单的 C 语言程序示例,包括打印 "Hello, World!"、计算两个数之和、交换两个变量值、检查字符是否为数字以及输入字符串并求长度:
1. **Hello, World!** (最简单的程序)
```c
#include <stdio.h>
int main() {
printf("Hello, World!\n");
return 0;
}
```
2. **加法计算器**
```c
#include <stdio.h>
int main() {
int num1, num2, sum;
printf("Enter two numbers: ");
scanf("%d %d", &num1, &num2);
sum = num1 + num2;
printf("Sum: %d\n", sum);
return 0;
}
```
3. **交换两个变量**
```c
#include <stdio.h>
void swap(int* a, int* b) {
int temp = *a;
*a = *b;
*b = temp;
}
int main() {
int x = 5, y = 10;
printf("Before swap: x=%d, y=%d\n", x, y);
swap(&x, &y);
printf("After swap: x=%d, y=%d\n", x, y);
return 0;
}
```
4. **判断字符是否为数字**
```c
#include <stdio.h>
char is_digit(char c) {
if(c >= '0' && c <= '9') {
return 1; // 返回非零值表示是数字
} else {
return 0;
}
}
int main() {
char ch;
printf("Enter a character: ");
scanf(" %c", &ch);
if(is_digit(ch)) {
printf("%c is a digit.\n", ch);
} else {
printf("%c is not a digit.\n", ch);
}
return 0;
}
```
5. **读取字符串并求长度**
```c
#include <stdio.h>
#include <string.h>
int main() {
char str[100];
printf("Enter a string: ");
fgets(str, sizeof(str), stdin);
str[strcspn(str, "\n")] = '\0'; // 清除换行符
int len = strlen(str);
printf("Length of the string: %d\n", len);
return 0;
}
```
阅读全文
相关推荐
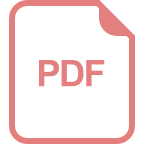
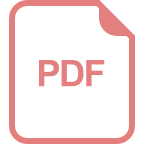
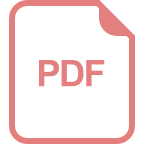


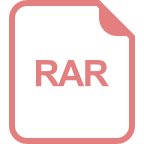


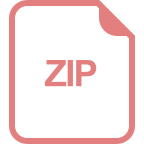
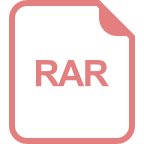
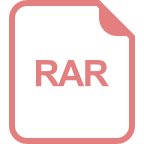
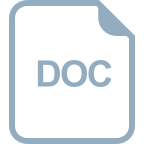
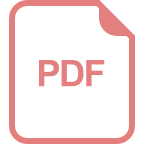
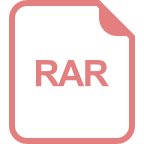
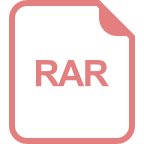
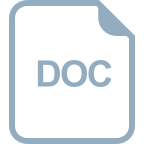
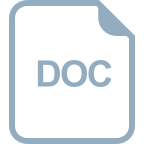
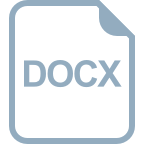
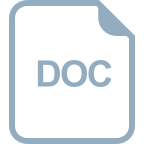