wpf点击按钮加载图片
时间: 2024-08-30 15:01:44 浏览: 61
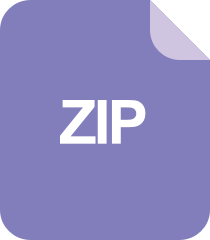
WPF 图片滑动轮播,按钮滑动,左右滑动,下标点击定位

在WPF(Windows Presentation Foundation)中,你可以使用`Button`控件配合`Image`控件以及事件处理来实现在点击按钮时动态加载图片。以下是一个基本的步骤:
1. 首先,在XAML文件中创建一个Button控件和一个Image控件:
```xml
<Button x:Name="LoadImageButton" Content="点击加载图片">
</Button>
<Image x:Name="MyImage" HorizontalAlignment="Center" VerticalAlignment="Center"/>
```
2. 然后,在对应的View.xaml.cs或ViewModel中,设置Button的Click事件处理器:
```csharp
private void LoadImageButton_Click(object sender, RoutedEventArgs e)
{
// 这里会是你的图片路径,可以是静态的也可以从数据源获取
string imagePath = "path_to_your_image.jpg";
// 使用Image.Source属性设置图片
MyImage.Source = new BitmapImage(new Uri(imagePath));
}
```
在这个例子中,当用户点击“点击加载图片”按钮时,Image控件的内容就会变为指定路径的图片。
阅读全文
相关推荐
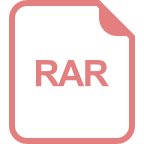

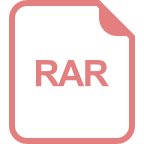
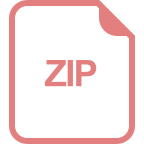
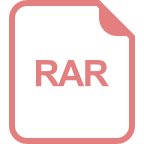



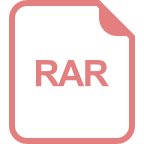
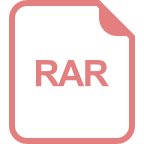
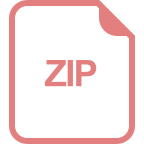
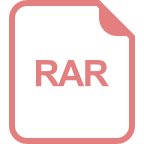
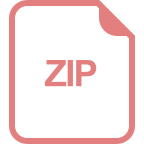



