帮我把代码写具体并能够输出
时间: 2024-12-10 12:53:58 浏览: 8
在C++中实现上述饭卡管理小程序的一个简单版本会包含以下核心代码片段。这里假设我们使用基本的控制台界面,并使用`iostream`库来进行输入输出。由于篇幅原因,只展示关键部分,实际项目会更复杂,包括更多的错误检查和异常处理。
**用户模块(User Module):**
```cpp
#include <iostream>
#include <string>
class User {
public:
User(std::string id, double balance)
: cardId(id), balance(balance) {}
void eat(int amount) {
if (balance >= amount) {
balance -= amount;
std::cout << "消费了" << amount << "元,当前余额:" << balance << std::endl;
} else {
std::cout << "余额不足!" << std::endl;
}
}
void checkBalance() {
std::cout << "当前余额:" << balance << std::endl;
}
void recharge(double newAmount) {
balance += newAmount;
std::cout << "已成功充值" << newAmount << "元,新的余额:" << balance << std::endl;
}
private:
std::string cardId;
double balance;
};
// 示例用户操作
int main() {
User user("123456", 100);
int choice;
while (true) {
std::cout << "请选择操作:1. 吃饭 2. 查看余额 3. 充值 4. 退出" << std::endl;
std::cin >> choice;
switch (choice) {
case 1: user.eat(50); break;
case 2: user.checkBalance(); break;
case 3: double amount;
std::cin >> amount;
user.recharge(amount); break;
case 4: return 0; // 退出循环
default: std::cout << "无效选择!" << std::endl;
}
}
}
```
**餐厅管理系统(Admin Module):**
```cpp
// Admin模块示例,未在此处展示,因为通常需要数据库连接或文件读写才能保存和检索用户数据
class Admin { ... }
// 这里仅用于演示如何从Admin获取信息,而非实现在用户模块中
void Admin::viewCardInfo(const std::string& cardId) {
// 在实际项目中,将查找数据库或文件中的用户记录
for (const auto& user : users) {
if (user.cardId == cardId) {
user.checkBalance();
break;
}
}
}
// 在主循环之外运行管理员功能
int main() { ... }
```
这部分代码是一个简化的版本,实际应用中还需要考虑更多的边界情况和用户交互。
阅读全文
相关推荐









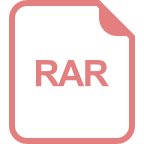


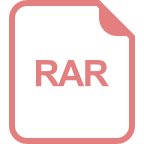





