netty中如何正确地将io线程池与业务线程池分开,请给出样例代码?业务线程池使用DefaultEventLoopGroup还是jdk中的普通线程池?这两者什么区别?
时间: 2024-12-07 09:12:22 浏览: 11
在Netty中,将I/O线程池与业务线程池分开是为了提高系统的性能和可扩展性。I/O线程池负责处理网络I/O操作,而业务线程池负责处理具体的业务逻辑。这样可以避免I/O操作阻塞业务逻辑的处理,反之亦然。
### 样例代码
以下是一个简单的Netty服务器示例,展示了如何将I/O线程池与业务线程池分开:
```java
import io.netty.bootstrap.ServerBootstrap;
import io.netty.channel.*;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.nio.NioServerSocketChannel;
import io.netty.channel.ChannelHandler.Sharable;
public class NettyServer {
private final int port;
public NettyServer(int port) {
this.port = port;
}
public void run() throws Exception {
// I/O线程池
EventLoopGroup bossGroup = new NioEventLoopGroup(1); // 负责接收连接的线程池
EventLoopGroup workerGroup = new NioEventLoopGroup(); // 负责处理连接的线程池
// 业务线程池
EventLoopGroup businessGroup = new DefaultEventLoopGroup(); // Netty提供的默认业务线程池
try {
ServerBootstrap b = new ServerBootstrap();
b.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new ChannelInitializer<Channel>() {
@Override
public void initChannel(Channel ch) {
ChannelPipeline p = ch.pipeline();
p.addLast(businessGroup, new BusinessHandler()); // 将业务处理添加到业务线程池
}
})
.option(ChannelOption.SO_BACKLOG, 128)
.childOption(ChannelOption.SO_KEEPALIVE, true);
ChannelFuture f = b.bind(port).sync();
f.channel().closeFuture().sync();
} finally {
workerGroup.shutdownGracefully();
bossGroup.shutdownGracefully();
businessGroup.shutdownGracefully();
}
}
public static void main(String[] args) throws Exception {
int port = 8080;
new NettyServer(port).run();
}
}
@Sharable
class BusinessHandler extends ChannelInboundHandlerAdapter {
@Override
public void channelRead(ChannelHandlerContext ctx, Object msg) {
// 业务处理逻辑
System.out.println("Received message: " + msg);
ctx.writeAndFlush(msg);
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) {
cause.printStackTrace();
ctx.close();
}
}
```
### 区别
1. **DefaultEventLoopGroup**:
- Netty提供的默认业务线程池实现。
- 适用于大多数业务场景。
- 线程数默认为CPU核心数的两倍。
2. **JDK中的普通线程池**:
- 例如,`ExecutorService`。
- 适用于需要更细粒度控制的场景。
- 可以根据需要自定义线程池参数。
### 总结
在Netty中,使用`DefaultEventLoopGroup`作为业务线程池是一个常见的选择,因为它与Netty的事件循环机制兼容良好,并且提供了良好的性能和可扩展性。如果你需要更细粒度的控制,可以选择使用JDK的普通线程池。
阅读全文
相关推荐
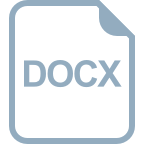
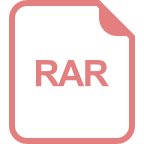


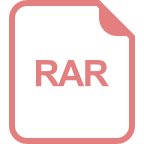
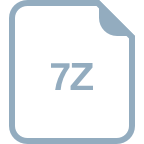
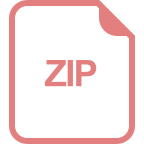
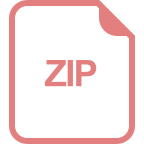
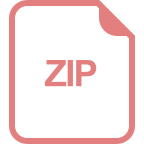
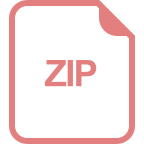
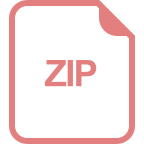
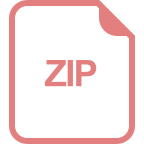
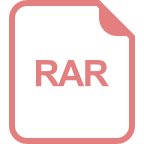
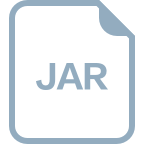
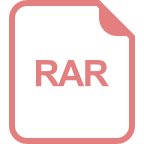
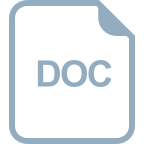