重构call apply bind方法
时间: 2024-02-15 07:59:59 浏览: 21
以下是重构call、apply、bind方法的示例:
1. 重构call方法:
```javascript
Function.prototype.myCall = function(context, ...args) {
context = context || window;
const fn = Symbol();
context[fn] = this;
const result = context[fn](...args);
delete context[fn];
return result;
};
// 示例
function greet(name) {
console.log(`Hello, ${name}!`);
}
const person = {
name: 'Alice'
};
greet.myCall(person, 'Bob'); // 输出:Hello, Bob!
```
2. 重构apply方法:
```javascript
Function.prototype.myApply = function(context, args) {
context = context || window;
const fn = Symbol();
context[fn] = this;
const result = context[fn](...args);
delete context[fn];
return result;
};
// 示例
function greet(name) {
console.log(`Hello, ${name}!`);
}
const person = {
name: 'Alice'
};
greet.myApply(person, ['Bob']); // 输出:Hello, Bob!
```
3. 重构bind方法:
```javascript
Function.prototype.myBind = function(context, ...args) {
const fn = this;
return function(...innerArgs) {
return fn.apply(context, [...args, ...innerArgs]);
};
};
// 示例
function greet(name) {
console.log(`Hello, ${name}!`);
}
const person = {
name: 'Alice'
};
const greetPerson = greet.myBind(person);
greetPerson('Bob'); // 输出:Hello, Bob!
```
相关推荐
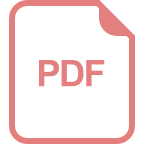
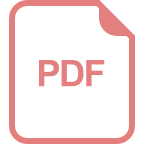
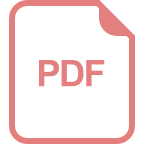














