生成一段以顺序存储方式实现栈的基本操作和数值转换的代码
时间: 2024-10-23 14:13:59 浏览: 23
在C++中,我们可以使用数组或动态数组(vector)来实现基本的栈数据结构。这里我们先用数组为例,然后进行栈的基本操作,如压入元素、弹出元素和查看顶部元素。至于数值转换,这里我们将演示如何将字符串转为整数并压入栈。
```cpp
#include <iostream>
#include <string>
using namespace std;
// 定义栈的大小和类型
#define STACK_SIZE 10
int stack[STACK_SIZE];
int top = -1; // 栈顶指针初始化为-1
// 压栈操作
void push(int value) {
if (top == STACK_SIZE - 1) {
cout << "Stack is full, cannot push." << endl;
return;
}
stack[++top] = value;
cout << "Pushed " << value << " to the stack." << endl;
}
// 弹栈操作
int pop() {
if (top == -1) {
cout << "Stack is empty, cannot pop." << endl;
return -1;
}
int value = stack[top--]; // 把顶部元素赋值给value,然后退栈
return value;
}
// 查看栈顶元素但不删除
int peek() {
if (top == -1) {
cout << "Stack is empty." << endl;
return -1;
}
return stack[top];
}
// 字符串转整数并压栈
void string_to_int(const string& str) {
try {
int num = stoi(str);
push(num);
cout << "Converted " << str << " to integer and pushed it: " << num << endl;
} catch (const invalid_argument& ia) {
cout << "Invalid input: could not convert to integer." << endl;
}
}
int main() {
// 初始化一个空栈
cout << "Initial stack: ";
for (int i = 0; i <= top; ++i)
cout << "-";
// 测试栈的操作
string_to_int("123");
string_to_int("abc"); // 这个字符串不能被转换为整数
// 随机添加和查看元素
push(456);
push('a');
cout << "Top element after pushing 'a': " << peek() << endl;
return 0;
}
```
阅读全文
相关推荐




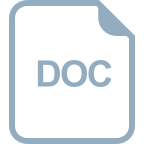
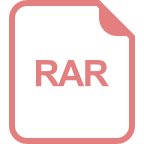

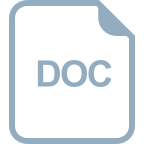










