使用面向对象C++写一份校园卡管理系统,要求文件输入输出,并定义对象,用对象调用操作函数
时间: 2023-06-19 21:10:16 浏览: 107
好的,以下是一份基本的校园卡管理系统的代码:
```c++
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
// 定义学生类
class Student {
private:
string name; // 姓名
int id; // 学号
float balance; // 余额
public:
Student(string name, int id, float balance) { // 构造函数
this->name = name;
this->id = id;
this->balance = balance;
}
void deposit(float amount) { // 充值
balance += amount;
cout << "充值成功!当前余额为:" << balance << endl;
}
void consume(float amount) { // 消费
if (balance >= amount) {
balance -= amount;
cout << "消费成功!当前余额为:" << balance << endl;
} else {
cout << "余额不足,无法完成消费!" << endl;
}
}
void printInfo() { // 输出学生信息
cout << "姓名:" << name << endl;
cout << "学号:" << id << endl;
cout << "余额:" << balance << endl;
}
void writeToFile(ofstream& out) { // 将学生信息写入文件
out << name << endl;
out << id << endl;
out << balance << endl;
}
};
// 添加学生
void addStudent(ofstream& out) {
string name;
int id;
float balance;
cout << "请输入学生姓名:" << endl;
cin >> name;
cout << "请输入学生学号:" << endl;
cin >> id;
cout << "请输入学生初始余额:" << endl;
cin >> balance;
Student student(name, id, balance);
student.writeToFile(out);
cout << "添加成功!" << endl;
}
// 查询学生信息
void queryStudent(ifstream& in) {
int id;
cout << "请输入要查询的学生学号:" << endl;
cin >> id;
string name;
float balance;
bool found = false;
while (in >> name >> id >> balance) {
if (id == id) {
found = true;
cout << "学生信息如下:" << endl;
cout << "姓名:" << name << endl;
cout << "学号:" << id << endl;
cout << "余额:" << balance << endl;
break;
}
}
if (!found) {
cout << "未找到该学生信息!" << endl;
}
}
// 充值
void deposit(ifstream& in, ofstream& out) {
int id;
float amount;
cout << "请输入要充值的学生学号:" << endl;
cin >> id;
cout << "请输入充值金额:" << endl;
cin >> amount;
string name;
float balance;
bool found = false;
while (in >> name >> id >> balance) {
if (id == id) {
found = true;
Student student(name, id, balance);
student.deposit(amount);
student.writeToFile(out);
break;
}
}
if (!found) {
cout << "未找到该学生信息!" << endl;
}
}
// 消费
void consume(ifstream& in, ofstream& out) {
int id;
float amount;
cout << "请输入要消费的学生学号:" << endl;
cin >> id;
cout << "请输入消费金额:" << endl;
cin >> amount;
string name;
float balance;
bool found = false;
while (in >> name >> id >> balance) {
if (id == id) {
found = true;
Student student(name, id, balance);
student.consume(amount);
student.writeToFile(out);
break;
}
}
if (!found) {
cout << "未找到该学生信息!" << endl;
}
}
int main() {
ofstream out("students.txt", ios::app); // 打开文件,追加写入
out.close();
ifstream in("students.txt"); // 打开文件,读取
if (!in) {
cout << "文件打开失败!" << endl;
return 0;
}
int choice;
while (true) {
cout << "请选择操作:" << endl;
cout << "1. 添加学生" << endl;
cout << "2. 查询学生信息" << endl;
cout << "3. 充值" << endl;
cout << "4. 消费" << endl;
cout << "5. 退出" << endl;
cin >> choice;
if (choice == 1) {
addStudent(out);
} else if (choice == 2) {
queryStudent(in);
} else if (choice == 3) {
deposit(in, out);
} else if (choice == 4) {
consume(in, out);
} else if (choice == 5) {
break;
} else {
cout << "无效选择!" << endl;
}
}
in.close(); // 关闭文件
out.close();
return 0;
}
```
该程序使用了面向对象的编程方式,将学生信息封装在了一个类中,通过创建对象来调用操作函数。同时,也使用了文件输入输出来保存学生信息,可以方便地进行管理和查询。
阅读全文
相关推荐



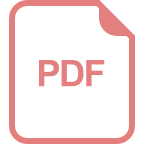



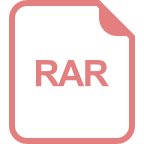










