写出代码,对excel文件制定的某一列,依据停用词.txt文件先去除停用词,然后对每一个单独进行词频统计,统计每一行出现的每个词的数量,最后将结果输出为excel
时间: 2024-05-01 22:22:57 浏览: 7
以下是使用Python实现的代码:
```python
import pandas as pd
import jieba
from collections import Counter
# 加载停用词
stopwords_path = "停用词.txt"
with open(stopwords_path, "r", encoding="utf-8") as f:
stopwords = set([line.strip() for line in f])
# 加载excel文件
excel_path = "数据.xlsx"
df = pd.read_excel(excel_path)
# 对指定列进行分词并去除停用词
def cut_words(text):
words = []
for word in jieba.cut(text):
if word not in stopwords:
words.append(word)
return words
# 统计词频
def count_words(words):
return dict(Counter(words))
# 对每一行进行词频统计
for index, row in df.iterrows():
text = str(row["文本"])
words = cut_words(text)
words_count = count_words(words)
for word, count in words_count.items():
df.at[index, word] = count
# 输出结果到excel
output_path = "结果.xlsx"
df.to_excel(output_path, index=False)
```
需要注意的是,以上代码使用了jieba分词库进行中文分词,需要先安装jieba库,可以使用以下命令进行安装:
```
pip install jieba
```
同时,需要将停用词保存为文本格式的文件,每个停用词占一行。以上代码默认停用词文件名为“停用词.txt”,需要根据实际情况进行修改。
相关推荐
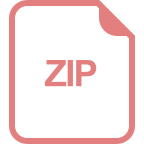














