uniapp在导航栏加入倒计时
时间: 2023-08-16 17:04:37 浏览: 156
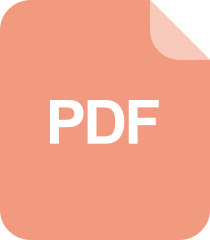
uni-app实现获取验证码倒计时功能
要在uniapp导航栏中加入倒计时,可以使用定时器和自定义导航栏组件来实现。
首先,在页面的onLoad函数中设置一个定时器,每隔一秒钟更新倒计时时间:
```javascript
onLoad: function () {
this.setData({
countdown: 60 // 设置倒计时时间
})
// 启动定时器
this.timer = setInterval(() => {
let countdown = this.data.countdown - 1
if (countdown < 0) {
clearInterval(this.timer)
return
}
this.setData({
countdown: countdown
})
}, 1000)
}
```
接着,在自定义导航栏组件中使用倒计时时间来显示倒计时:
```javascript
<template>
<view class="custom-navbar">
<view class="title">{{title}}</view>
<view class="countdown" v-if="countdown >= 0">{{countdown}}s</view>
</view>
</template>
<script>
export default {
name: 'CustomNavbar',
props: {
title: {
type: String,
default: ''
},
countdown: {
type: Number,
default: -1
}
}
}
</script>
<style>
.custom-navbar {
display: flex;
justify-content: space-between;
align-items: center;
height: 44px;
padding: 0 15px;
background-color: #fff;
box-shadow: 0 1px 3px rgba(0, 0, 0, 0.2);
}
.title {
font-size: 16px;
font-weight: bold;
}
.countdown {
font-size: 14px;
color: #666;
}
</style>
```
最后,在页面中使用自定义导航栏组件,并将倒计时时间传递给组件:
```javascript
<template>
<view class="container">
<custom-navbar title="页面标题" :countdown="countdown" />
<!-- 页面内容 -->
</view>
</template>
<script>
import CustomNavbar from '@/components/CustomNavbar'
export default {
components: {
CustomNavbar
},
data () {
return {
countdown: 60
}
},
onLoad: function () {
// 启动定时器
this.timer = setInterval(() => {
let countdown = this.countdown - 1
if (countdown < 0) {
clearInterval(this.timer)
return
}
this.countdown = countdown
}, 1000)
}
}
</script>
```
这样,在导航栏中就可以显示倒计时了。需要注意的是,在页面销毁时要清除定时器,否则会导致内存泄漏。可以在onUnload函数中清除定时器:
```javascript
onUnload: function () {
clearInterval(this.timer)
}
```
阅读全文
相关推荐
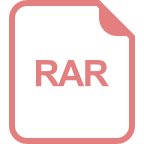
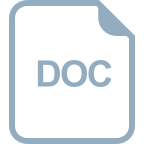













