typedef struct { QElemType base[MAXQSIZE]; int front; int rear; } SqQueue; 怎么判断数组是否创建成功
时间: 2024-02-15 10:44:15 浏览: 71
在 C 语言中,使用结构体定义队列时,队列的底层数据结构一般是使用数组实现的。在结构体中,使用了一个 `base` 数组来存储队列中的元素,而 MAXQSIZE 表示了数组的最大容量。因此,可以通过以下方式判断队列数组是否创建成功:
1. 首先,需要在程序中创建一个 `SqQueue` 类型的变量,例如 `SqQueue myQueue;`。
2. 然后,可以通过 `sizeof(SqQueue)` 来获取 `SqQueue` 结构体占用的字节数。如果字节数为 `MAXQSIZE * sizeof(QElemType) + 2 * sizeof(int)`,则说明 `base` 数组创建成功。
3. 可以使用 `myQueue.front` 和 `myQueue.rear` 来判断队列是否创建成功。如果 `front` 和 `rear` 的值都为 0,说明队列创建成功。
需要注意的是,在 C 语言中,数组是静态分配的,一旦定义了数组大小,就无法再修改。因此,在创建队列时必须确定队列的最大容量,以避免溢出。
相关问题
#include<iostream> using namespace std; #define MAXQSIZE 100 #define OK 1 #define ERROR 0 #define OVERFLOW -2 typedef char QElemType; typedef char SElemType; typedef int Status; typedef struct { QElemType *base; int front; int rear; } SqQueue; Status InitQueue(SqQueue &Q) { ; if (!Q.base) exit(OVERFLOW); ; return OK; } Status EnQueue(SqQueue &Q, QElemType e) { if ( ) return ERROR; Q.base[Q.rear] = e; ; return OK; } Status DeQueue(SqQueue &Q, QElemType &e) { if ( ) return ERROR; e = Q.base[Q.front]; ; return OK; } int main() { SqQueue Q; int n, i; char c; InitQueue(Q); cin >> n; for(i=0;i<n;i++){ cin >> c; EnQueue(Q,c); } for(i=0;i<n;i++){ DeQueue(Q,c); cout << c << " "; } return 0; }
这段代码实现了一个基于数组的队列,可以将字符元素入队和出队,并按照入队顺序输出。
其中,SqQueue是一个结构体,包含了队列的基地址、队头指针和队尾指针。
函数InitQueue用来初始化队列,Q.base是队列的基地址,通过动态分配内存来实现队列的创建。
函数EnQueue用来将元素e入队,如果队列已满,则返回ERROR;否则将元素e放入队尾,并将队尾指针后移一位。
函数DeQueue用来将队头元素出队,并将其存储到变量e中,如果队列为空,则返回ERROR;否则将队头元素取出,并将队头指针后移一位。
在主函数中,先初始化一个空队列,输入n个字符元素并依次入队,再依次出队并输出。
利用队列操作,模拟实现打印作业任务管理。 #define ERROR 1 #define OK 0 #define OVERFLOW 1 typedef int QElemType; typedef int Status; #define MAXQSIZE 100 //最大队列长度 typedef struct { QElemType *base; // 动态分配存储空间 int front; // 头指针,若队列不空,指向队列头元素 int rear; // 尾指针,若队列不空, //指向队列尾元素的下一个位置 }SqQueue;
以下是模拟实现打印作业任务管理的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define ERROR 1
#define OK 0
#define OVERFLOW 1
typedef int QElemType;
typedef int Status;
#define MAXQSIZE 100 //最大队列长度
typedef struct {
QElemType *base; // 动态分配存储空间
int front; // 头指针,若队列不空,指向队列头元素
int rear; // 尾指针,若队列不空,
//指向队列尾元素的下一个位置
} SqQueue;
Status InitQueue(SqQueue *Q) {
Q->base = (QElemType*)malloc(MAXQSIZE * sizeof(QElemType));
if (!Q->base) exit(OVERFLOW); //存储分配失败
Q->front = Q->rear = 0;
return OK;
}
int QueueLength(SqQueue Q) {
return (Q.rear - Q.front + MAXQSIZE) % MAXQSIZE;
}
Status EnQueue(SqQueue *Q, QElemType e) {
if ((Q->rear + 1) % MAXQSIZE == Q->front) return ERROR; //队列满
Q->base[Q->rear] = e;
Q->rear = (Q->rear + 1) % MAXQSIZE;
return OK;
}
Status DeQueue(SqQueue *Q, QElemType *e) {
if (Q->front == Q->rear) return ERROR; //队列空
*e = Q->base[Q->front];
Q->front = (Q->front + 1) % MAXQSIZE;
return OK;
}
Status PrintQueue(SqQueue Q) {
if (Q.front == Q.rear) {
printf("队列为空!\n");
return OK;
}
printf("队列中的元素为:");
int i = Q.front;
while (i != Q.rear) {
printf("%d ", Q.base[i]);
i = (i + 1) % MAXQSIZE;
}
printf("\n");
return OK;
}
int main() {
SqQueue Q;
QElemType e;
InitQueue(&Q);
printf("请输入打印作业的数量:");
int n;
scanf("%d", &n);
for (int i = 1; i <= n; i++) {
int job;
printf("请输入第%d个打印作业需要的打印时间:", i);
scanf("%d", &job);
if (EnQueue(&Q, job) == ERROR) {
printf("队列已满,打印作业%d无法加入队列!\n", i);
}
}
printf("\n打印作业队列中共有%d个打印作业。\n", QueueLength(Q));
PrintQueue(Q);
printf("\n开始打印作业...\n");
while (Q.front != Q.rear) {
DeQueue(&Q, &e);
printf("打印作业%d已完成打印,剩余打印作业数量:%d\n", e, QueueLength(Q));
}
printf("所有打印作业已完成打印。\n");
return 0;
}
```
以上程序实现了一个打印作业任务管理的队列,用户可以输入打印作业的数量和每个打印作业需要的打印时间,程序将打印作业加入队列,并依次完成打印作业。在队列满的情况下,无法将新的打印作业加入队列,并输出相应的提示信息。
阅读全文
相关推荐
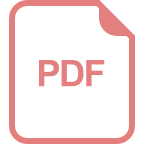



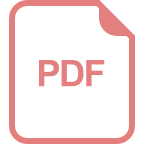
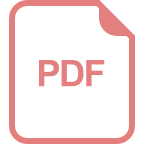
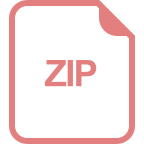