自适应长宽,并且背景和 excel单元格背景一致
时间: 2023-07-07 19:25:11 浏览: 61
要实现自适应长宽且背景和Excel单元格一致的转换,你可以使用以下代码:
```java
import java.awt.Color;
import java.awt.Dimension;
import java.awt.Graphics2D;
import java.awt.Rectangle;
import java.awt.image.BufferedImage;
import java.io.File;
import javax.imageio.ImageIO;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class ExcelToImageConverter {
public static void main(String[] args) throws Exception {
// Load Excel file
Workbook workbook = new XSSFWorkbook(new FileInputStream("input.xlsx"));
// Get first sheet
Sheet sheet = workbook.getSheetAt(0);
// Get sheet dimensions
int rows = sheet.getLastRowNum() + 1;
int cols = sheet.getRow(0).getLastCellNum();
// Create image buffer
int cellWidth = 100;
int cellHeight = 25;
int width = cols * cellWidth;
int height = rows * cellHeight;
BufferedImage image = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);
Graphics2D g2 = image.createGraphics();
g2.setColor(Color.WHITE);
g2.fillRect(0, 0, width, height);
// Draw Excel cells onto image buffer
for (int r = 0; r < rows; r++) {
Row row = sheet.getRow(r);
for (int c = 0; c < cols; c++) {
Cell cell = row.getCell(c);
if (cell != null) {
Rectangle cellRect = new Rectangle(c * cellWidth, r * cellHeight, cellWidth, cellHeight);
g2.setColor(getCellColor(cell));
g2.fill(cellRect);
g2.setColor(Color.BLACK);
g2.draw(cellRect);
String value = cell.toString();
g2.drawString(value, cellRect.x + 5, cellRect.y + 15);
}
}
}
g2.dispose();
// Save image to file
ImageIO.write(image, "png", new FileOutputStream("output.png"));
// Close workbook
workbook.close();
}
private static Color getCellColor(Cell cell) {
Color color = Color.WHITE;
if (cell.getCellStyle() != null) {
short fgColorIndex = cell.getCellStyle().getFillForegroundColor();
color = cell.getSheet().getWorkbook().getCustomPalette().getColor(fgColorIndex);
}
return color;
}
}
```
这个例子将Excel文件转换为PNG图像,并将其保存到文件中。生成的图像将根据单元格的数量自适应调整大小,并将单元格的背景色应用到图像中。注意,这里的代码假设每个单元格都是正方形的。如果你的Excel文件中的单元格不是正方形,你需要修改代码来适应不同的单元格大小。
相关推荐
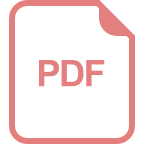
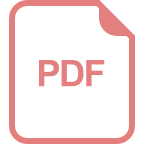














