c++/c 语言构建循环链表
时间: 2023-09-12 14:08:42 浏览: 47
循环链表和普通链表的区别在于,循环链表的尾节点指针指向头节点,形成一个环。下面是用C++语言构建循环链表的示例代码:
```c++
#include <iostream>
using namespace std;
// 定义链表节点
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(NULL) {}
};
// 构建循环链表
ListNode* buildCycleList(int n) {
ListNode* head = new ListNode(1);
ListNode* cur = head;
for (int i = 2; i <= n; i++) {
cur->next = new ListNode(i);
cur = cur->next;
}
cur->next = head; // 将尾节点指针指向头节点,形成环
return head;
}
// 打印循环链表
void printCycleList(ListNode* head, int n) {
ListNode* cur = head;
for (int i = 0; i < n; i++) {
cout << cur->val << " ";
cur = cur->next;
}
cout << endl;
}
int main() {
int n = 5;
ListNode* head = buildCycleList(n);
printCycleList(head, n);
return 0;
}
```
以上代码中,`buildCycleList`函数用于构建循环链表,`printCycleList`函数用于打印循环链表。主函数中,我们构建了一个包含5个节点的循环链表,并输出链表中每个节点的值。
相关推荐
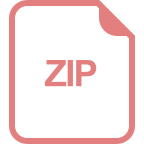
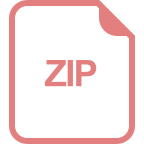
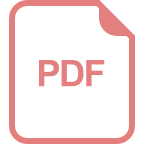














