如何设置串口以允许中断?
时间: 2024-09-08 21:04:34 浏览: 59
在计算机系统中,设置串口以允许中断通常涉及到串口控制器的配置,以便它可以在接收到数据时发出中断信号。在不同的操作系统和编程环境中,这一过程可能会有所不同。以下是一个通用的概述:
1. 确定串口号:首先,你需要确定你的设备连接到哪个串口(例如COM1, COM2等)。
2. 检查硬件支持:确保你的串口硬件支持中断驱动的数据传输。
3. 配置串口参数:使用适当的工具或编程接口(如Windows的DeviceIoControl函数或Linux的termios结构)设置串口参数。这些参数包括波特率、数据位、停止位、校验位等。
4. 启用中断:在串口设置中找到与中断相关的选项,通常是在串口控制器的配置寄存器中设置相应的位。这可能包括启用接收中断或发送中断等。
5. 编写中断服务例程(ISR):如果是在编程层面处理中断,你需要编写一个中断服务例程来响应串口的中断信号,并处理接收到的数据。
6. 启动串口:最后,启动串口,此时它应该能够接收数据并根据设置发出中断信号。
以下是在Linux系统中使用termios结构配置串口并启用中断接收数据的一个简单示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
#include <errno.h>
int set_interface_attribs(int fd, int speed, int parity) {
struct termios tty;
memset(&tty, 0, sizeof tty);
if (tcgetattr(fd, &tty) != 0) {
printf("error %d from tcgetattr", errno);
return -1;
}
cfsetospeed(&tty, speed);
cfsetispeed(&tty, speed);
tty.c_cflag = (tty.c_cflag & ~CSIZE) | CS8; // 8-bit chars
// disable IGNBRK for mismatched speed tests; otherwise receive break
// as \000 chars
tty.c_iflag &= ~IGNBRK; // disable break processing
tty.c_lflag = 0; // no signaling chars, no echo,
// no canonical processing
tty.c_oflag = 0; // no remapping, no delays
tty.c_cc[VMIN] = 0; // read doesn't block
tty.c_cc[VTIME] = 5; // 0.5 seconds read timeout
tty.c_iflag &= ~(IXON | IXOFF | IXANY); // shut off xon/xoff ctrl
tty.c_cflag |= (CLOCAL | CREAD); // ignore modem controls,
// enable reading
tty.c_cflag &= ~(PARENB | PARODD); // shut off parity
tty.c_cflag |= parity;
tty.c_cflag &= ~CSTOPB;
tty.c_cflag &= ~CRTSCTS;
if (tcsetattr(fd, TCSANOW, &tty) != 0) {
printf("error %d from tcsetattr", errno);
return -1;
}
return 0;
}
int main() {
int serial_port = open("/dev/ttyS0", O_RDWR);
if (serial_port < 0) {
printf("Error %i from open: %s\n", errno, strerror(errno));
return 1;
}
// 设置串口参数
if (set_interface_attribs(serial_port, B9600, 0) < 0) {
close(serial_port);
return 2;
}
// 启用中断(具体方法取决于硬件和操作系统)
// ...
return 0;
}
```
这个代码示例仅提供了一个基本的设置过程,实际上在启用中断时可能需要访问更底层的硬件寄存器或使用特定的系统调用,这依赖于你的硬件和操作系统。
阅读全文
相关推荐














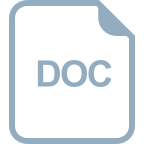


