Java解压zip并上传服务器
时间: 2023-05-17 19:05:17 浏览: 75
可以使用Java的ZipInputStream和FileOutputStream类来解压zip文件,并使用Java的HttpURLConnection和OutputStream类将解压后的文件上传到服务器。以下是示例代码:
```
import java.io.*;
import java.net.HttpURLConnection;
import java.net.URL;
import java.util.zip.ZipEntry;
import java.util.zip.ZipInputStream;
public class ZipUploader {
public static void main(String[] args) throws Exception {
String zipFilePath = "path/to/zip/file.zip";
String serverUrl = "http://example.com/upload";
// Open the zip file
ZipInputStream zipInputStream = new ZipInputStream(new FileInputStream(zipFilePath));
ZipEntry zipEntry;
// Loop through all the entries in the zip file
while ((zipEntry = zipInputStream.getNextEntry()) != null) {
// Extract the entry to a temporary file
File tempFile = File.createTempFile(zipEntry.getName(), null);
FileOutputStream fileOutputStream = new FileOutputStream(tempFile);
byte[] buffer = new byte[1024];
int bytesRead;
while ((bytesRead = zipInputStream.read(buffer)) != -1) {
fileOutputStream.write(buffer, 0, bytesRead);
}
fileOutputStream.close();
// Upload the temporary file to the server
URL url = new URL(serverUrl);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("POST");
connection.setDoOutput(true);
OutputStream outputStream = connection.getOutputStream();
FileInputStream fileInputStream = new FileInputStream(tempFile);
while ((bytesRead = fileInputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, bytesRead);
}
fileInputStream.close();
outputStream.close();
// Delete the temporary file
tempFile.delete();
}
// Close the zip file
zipInputStream.close();
}
}
```
相关推荐
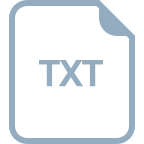














