基于遗传算法的自动组卷系统代码
时间: 2023-09-14 19:06:33 浏览: 242
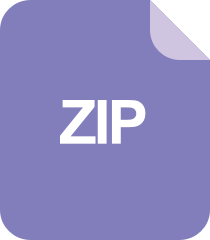
java_遗传算法_

遗传算法是一种优化算法,可以用于自动组卷系统。以下是一个基于遗传算法的自动组卷系统的代码示例:
```python
import random
class Exam:
def __init__(self, questions, num_questions, exam_length):
self.questions = questions
self.num_questions = num_questions
self.exam_length = exam_length
self.fitness = 0
def __repr__(self):
return f"Exam - Fitness: {self.fitness}, Questions: {self.questions}"
class GeneticAlgorithm:
def __init__(self, population_size, mutation_rate, crossover_rate, elitism):
self.population_size = population_size
self.mutation_rate = mutation_rate
self.crossover_rate = crossover_rate
self.elitism = elitism
def init_population(self, num_questions, exam_length):
population = []
for i in range(self.population_size):
questions = random.sample(range(num_questions), exam_length)
exam = Exam(questions, num_questions, exam_length)
population.append(exam)
return population
def evaluate_fitness(self, population):
for exam in population:
fitness = 0
for i in range(exam.num_questions):
if i in exam.questions:
fitness += 1
exam.fitness = fitness
def select_parents(self, population):
parents = []
for i in range(self.elitism):
parents.append(max(population, key=lambda x: x.fitness))
for i in range(self.population_size - self.elitism):
parent1 = random.choice(population)
parent2 = random.choice(population)
if parent1.fitness > parent2.fitness:
parents.append(parent1)
else:
parents.append(parent2)
return parents
def crossover(self, parents):
children = []
for i in range(0, len(parents), 2):
parent1 = parents[i]
parent2 = parents[i+1]
if random.random() < self.crossover_rate:
crossover_point = random.randint(1, parent1.exam_length - 1)
child1 = Exam(parent1.questions[:crossover_point] + parent2.questions[crossover_point:], parent1.num_questions, parent1.exam_length)
child2 = Exam(parent2.questions[:crossover_point] + parent1.questions[crossover_point:], parent2.num_questions, parent2.exam_length)
children.append(child1)
children.append(child2)
else:
children.append(parent1)
children.append(parent2)
return children
def mutate(self, population):
for exam in population:
for i in range(exam.exam_length):
if random.random() < self.mutation_rate:
exam.questions[i] = random.randint(0, exam.num_questions - 1)
def evolve_population(self, population):
parents = self.select_parents(population)
children = self.crossover(parents)
self.mutate(children)
population = parents + children
self.evaluate_fitness(population)
return population
def get_best(self, population):
return max(population, key=lambda x: x.fitness)
# Example usage
genetic_algorithm = GeneticAlgorithm(population_size=100, mutation_rate=0.01, crossover_rate=0.7, elitism=2)
population = genetic_algorithm.init_population(num_questions=50, exam_length=10)
genetic_algorithm.evaluate_fitness(population)
best_exam = None
for i in range(100):
population = genetic_algorithm.evolve_population(population)
best_exam = genetic_algorithm.get_best(population)
print(f"Generation {i+1}: {best_exam}")
```
这个代码实现了一个自动组卷系统,使用遗传算法优化试卷的组成。在初始化时,我们随机生成一定数量的试卷,并使用评估函数计算它们的适应度。然后,我们使用选择、交叉和变异操作来进化试卷的种群。在每个进化迭代中,我们根据适应度评估种群,并选择最佳试卷。最终,我们得到了最好的试卷。
阅读全文
相关推荐
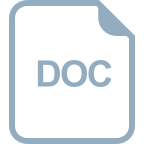
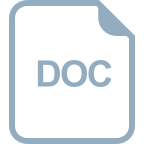
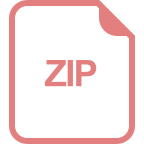
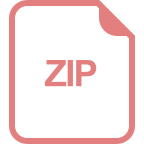
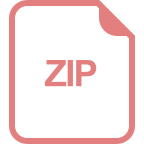
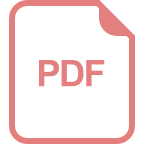
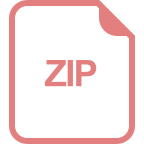
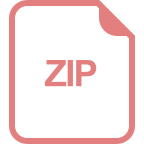
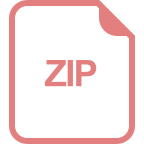
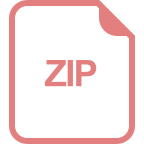
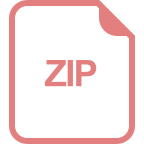
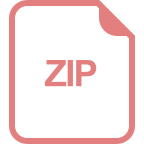
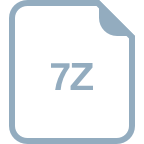
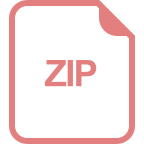
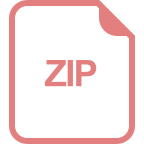
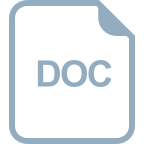
