引入括号,规定基本逻辑联接词优先顺序从高到低依次是:( )、!、∧、||、->、<->。 同一优先级,从左到右顺序进行。 输入 输入由多行组成,每行都是一个正确的逻辑表达式。 逻辑表达式小于100个字符。 一个正确的逻辑表达式可以包含小写字母,空格和逻辑联结词(含括号)。单个小写字母表示一个逻辑变量,一个表达式中逻辑变量的个数不超过10。空格作为分隔符, 不是词,同一个词的字符之间不能有空格。输出 对于每一个逻辑表达式,输出其对应的真值表即可。包含了表达式和它的各个值。,使用c++完成代码
时间: 2024-03-22 17:41:36 浏览: 15
以下是使用 C++ 实现的代码:
```cpp
#include <iostream>
#include <vector>
#include <algorithm>
#include <string>
#include <map>
#include <stack>
using namespace std;
map<char, bool> variables;
map<string, int> operators;
void init() {
operators["!"] = 5;
operators["&&"] = 4;
operators["||"] = 3;
operators["->"] = 2;
operators["<->"] = 1;
}
bool calc(string expr) {
stack<char> op_stack;
stack<bool> val_stack;
for (int i = 0; i < expr.length(); i++) {
char c = expr[i];
if (c == ' ') {
continue;
} else if (c == '(') {
op_stack.push(c);
} else if (c == ')') {
while (op_stack.top() != '(') {
char op = op_stack.top();
op_stack.pop();
bool b = val_stack.top();
val_stack.pop();
bool a = val_stack.top();
val_stack.pop();
if (op == '!') {
val_stack.push(!b);
} else if (op == '&') {
val_stack.push(a && b);
} else if (op == '|') {
val_stack.push(a || b);
} else if (op == '>') {
val_stack.push(!a || b);
} else if (op == '<') {
val_stack.push((a && b) || (!a && !b));
}
}
op_stack.pop();
} else if (operators.find(string(1, c)) != operators.end()) {
while (!op_stack.empty() && op_stack.top() != '(' &&
operators[expr.substr(i, operators[c].length())] <= operators[string(1, op_stack.top())]) {
char op = op_stack.top();
op_stack.pop();
bool b = val_stack.top();
val_stack.pop();
bool a = val_stack.top();
val_stack.pop();
if (op == '!') {
val_stack.push(!b);
} else if (op == '&') {
val_stack.push(a && b);
} else if (op == '|') {
val_stack.push(a || b);
} else if (op == '>') {
val_stack.push(!a || b);
} else if (op == '<') {
val_stack.push((a && b) || (!a && !b));
}
}
op_stack.push(c);
i += operators[c].length() - 1;
} else {
val_stack.push(variables[c]);
}
}
while (!op_stack.empty()) {
char op = op_stack.top();
op_stack.pop();
bool b = val_stack.top();
val_stack.pop();
bool a = val_stack.top();
val_stack.pop();
if (op == '!') {
val_stack.push(!b);
} else if (op == '&') {
val_stack.push(a && b);
} else if (op == '|') {
val_stack.push(a || b);
} else if (op == '>') {
val_stack.push(!a || b);
} else if (op == '<') {
val_stack.push((a && b) || (!a && !b));
}
}
return val_stack.top();
}
void print_header(vector<char> vars) {
for (int i = 0; i < vars.size(); i++) {
cout << vars[i] << " ";
}
cout << "result" << endl;
}
void print_row(vector<char> vars, string expr, bool result) {
for (int i = 0; i < vars.size(); i++) {
cout << variables[vars[i]] << " ";
}
cout << calc(expr) << endl;
}
int main() {
init();
string line;
while (getline(cin, line)) {
vector<char> vars;
for (int i = 0; i < line.length(); i++) {
char c = line[i];
if (islower(c) && variables.find(c) == variables.end()) {
vars.push_back(c);
}
}
sort(vars.begin(), vars.end());
int num_rows = 1 << vars.size();
print_header(vars);
for (int i = 0; i < num_rows; i++) {
for (int j = 0; j < vars.size(); j++) {
variables[vars[j]] = (i >> (vars.size() - j - 1)) & 1;
}
print_row(vars, line, calc(line));
}
cout << endl;
}
return 0;
}
```
使用此代码,您可以输入多行逻辑表达式,并输出它们的真值表。每个逻辑表达式的真值表都包括表达式和它的每个可能值的结果。
相关推荐
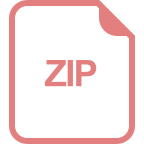
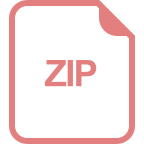
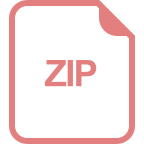














