mybatis-plus配置mapper.xml
时间: 2023-05-31 12:18:00 浏览: 737
### 回答1:
Mybatis-Plus是Mybatis的增强工具,它可以简化Mybatis的开发流程,提高开发效率。在使用Mybatis-Plus时,我们需要配置mapper.xml文件,这个文件是用来映射数据库表和Java对象的。
在配置mapper.xml文件时,我们需要定义SQL语句和参数映射关系。具体来说,我们需要定义select、insert、update和delete等操作的SQL语句,并且将参数映射到SQL语句中。此外,我们还需要定义resultMap,用来将查询结果映射到Java对象中。
在Mybatis-Plus中,我们可以使用注解来代替mapper.xml文件,这样可以更加简化开发流程。但是,在一些复杂的场景下,仍然需要使用mapper.xml文件来进行配置。
### 回答2:
Mybatis-plus是Mybatis的增强工具,在Mybatis的基础上扩展了更多的功能。它的使用相对简单,通过一些配置可以实现快速开发。
mybatis-plus配置mapper.xml主要有以下几步:
1.引入依赖
在pom.xml中加入如下依赖
```
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.x.x</version>
</dependency>
```
2.配置数据源
在application.properties中添加数据库连接信息
```
spring.datasource.driver-class-name=com.mysql.jdbc.Driver
spring.datasource.url=jdbc:mysql://localhost:3306/test?useSSL=false&useUnicode=true&characterEncoding=UTF-8&serverTimezone=UTC
spring.datasource.username=root
spring.datasource.password=root
```
3.配置mapper.xml
在resources/mapper目录下,创建mapper.xml文件,如UserMapper.xml,并在application.properties中添加mapper文件的路径
```
mybatis-plus.mapper-locations=classpath:mapper/*Mapper.xml
```
在mapper.xml文件中,使用mybatis-plus提供的标签来进行增删改查操作。
例如:
```
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd" >
<mapper namespace="com.example.demo.dao.UserMapper">
<select id="selectById" resultType="com.example.demo.entity.User">
select * from user where id=#{id}
</select>
<select id="selectAll" resultType="com.example.demo.entity.User">
select * from user
</select>
<insert id="insert" parameterType="com.example.demo.entity.User">
insert into user(name,age) values(#{name},#{age})
</insert>
<update id="updateById" parameterType="com.example.demo.entity.User">
update user set name=#{name},age=#{age} where id=#{id}
</update>
<delete id="deleteById" parameterType="int">
delete from user where id=#{id}
</delete>
</mapper>
```
4.编写实体类
mybatis-plus使用实体类来完成对象关系映射,所以需要编写实体类,例如:
```
@Data
public class User {
private Integer id;
private String name;
private Integer age;
}
```
实体类需要添加@Data注解以及getter和setter方法。
5.编写Mapper接口
创建UserMapper接口,并继承BaseMapper接口,例如:
```
public interface UserMapper extends BaseMapper<User> {
}
```
BaseMapper类提供了基本的增删改查接口。
6.使用mapper
在service层注入UserMapper,可以使用mapper中提供的方法,例如:
```
@Service
public class UserServiceImpl implements UserService {
@Autowired
private UserMapper userMapper;
@Override
public User selectById(Integer id) {
return userMapper.selectById(id);
}
@Override
public List<User> selectAll() {
return userMapper.selectList(null);
}
@Override
public int insert(User user) {
return userMapper.insert(user);
}
@Override
public int updateById(User user) {
return userMapper.updateById(user);
}
@Override
public int deleteById(Integer id) {
return userMapper.deleteById(id);
}
}
```
通过以上步骤,就可以完成mybatis-plus的mapper.xml配置。
### 回答3:
Mybatis-Plus 是一款基于 Mybatis 对其进行了增强的工具,通过简化 Mybatis 的配置,提供了许多方便实用的功能,如自动生成代码、分页插件、多租户支持等。
在 Mybatis 中,Mapper 配置文件是定义 SQL 的地方。在 Mybatis-Plus 中也有相应的配置文件,称为 Mapper XML,它是直接使用 Mybatis 的 Mapper 配置文件,只是在此基础上增加了一些关键字和标签用于支持额外的功能。Mapper XML 的使用方式与 Mybatis 的 Mapper 配置文件一样,只是包含了更多的功能。
在 Mybatis-Plus 中使用 Mapper XML 首先需要在项目中配置 Mybatis-Plus,在 pom.xml 文件中添加对 Mybatis-Plus 的依赖。然后在 Spring Boot 中的配置文件 application.yml 中添加 Mybatis-Plus 的配置项,如下所示:
```
mybatis-plus:
mapper-locations: classpath:/mappers/**/*.xml # Mapper XML 文件所在目录
```
其中,mapper-locations 配置项指定了 Mapper XML 文件所在目录,可以通过通配符 ** 来匹配所有子目录中的 Mapper XML 文件。在项目中,可以将 Mapper XML 文件放到 resources/mappers 目录下,然后在 Mybatis-Plus 的配置项中指定即可。
在 Mapper XML 文件中,我们可以定义 SQL 语句,并使用 Mybatis-Plus 提供的关键字和标签来增强 SQL 的功能。例如,我们可以使用 select 标签定义一个查询 SQL 语句,使用 where 标签定义查询条件:
```
<select id="selectById" resultType="Blog">
select * from blog
where id = #{id}
</select>
```
在 Mapper XML 中,我们还可以使用 Mybatis-Plus 提供的关键字和标签来实现更多的功能,例如分页插件、自定义 SQL 语句等。使用 Mybatis-Plus 配置 Mapper XML 可以大大简化 SQL 的编写,提高代码的可维护性。
阅读全文
相关推荐
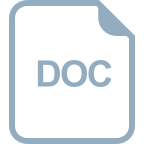
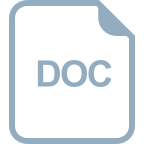
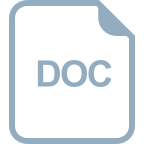
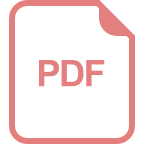














