定义一个字符串数组要求在数组中查找从键盘输入一个字符出现的次数
时间: 2023-05-16 21:06:03 浏览: 57
可以使用以下代码实现:
#include <stdio.h>
#include <string.h>
int main() {
char str[100];
char c;
int count = 0;
printf("请输入一个字符串:");
scanf("%s", str);
printf("请输入要查找的字符:");
scanf(" %c", &c);
for (int i = 0; i < strlen(str); i++) {
if (str[i] == c) {
count++;
}
}
printf("字符 %c 出现的次数为 %d\n", c, count);
return 0;
}
相关问题
在C语言中,定义一个字符串数组用于存放若干单词,从键盘输入某个单词,判断是否已存在于单词数组中
可以使用strcmp()函数来比较输入的单词和数组中的单词是否相同。具体实现可以参考以下示例代码:
```c
#include <stdio.h>
#include <string.h>
#define MAX_WORDS 100 // 定义单词数组的最大容量
#define MAX_LENGTH 50 // 定义单词的最大长度
int main() {
char words[MAX_WORDS][MAX_LENGTH]; // 定义单词数组
int count = 0; // 已存储的单词数量
char input[MAX_LENGTH]; // 存储从键盘输入的单词
// 从键盘输入若干个单词,直到输入exit为止
printf("请输入单词(输入exit结束):\n");
while (1) {
scanf("%s", words[count]);
if (strcmp(words[count], "exit") == 0) {
break;
}
count++;
}
// 从键盘输入要查找的单词
printf("请输入要查找的单词:\n");
scanf("%s", input);
// 在单词数组中查找输入的单词
int i;
for (i = 0; i < count; i++) {
if (strcmp(words[i], input) == 0) {
printf("单词 \"%s\" 已存在于数组中。\n", input);
break;
}
}
if (i == count) {
printf("单词 \"%s\" 不在数组中。\n", input);
}
return 0;
}
```
在上述代码中,我们定义了一个字符串数组words来存储输入的单词,同时定义了变量count来记录已存储的单词数量。在输入单词时,我们使用while循环不断从键盘读取输入,直到输入exit为止。在查找单词时,我们使用for循环遍历数组中的单词,如果找到了输入的单词,则输出相应的提示信息,否则输出不存在的提示信息。
创建一个字符数组,从键盘输入一个字符串保存到字符串数组中。编程输出字符“i”在数组中最后一次出现的位置
下面是一个 C++ 代码示例,可以实现你的需求:
```cpp
#include <iostream>
#include <cstring>
using namespace std;
int main() {
const int MAX_LEN = 100;
char str[MAX_LEN + 1];
cin.getline(str, MAX_LEN + 1); // 从键盘读入字符串
int last_i_pos = -1;
int len = strlen(str);
for (int i = 0; i < len; i++) {
if (str[i] == 'i') {
last_i_pos = i;
}
}
if (last_i_pos >= 0) {
cout << "字符'i'在数组中最后一次出现的位置是:" << last_i_pos << endl;
} else {
cout << "数组中没有出现字符'i'" << endl;
}
return 0;
}
```
这段代码首先定义了一个字符数组 `str`,并且将从键盘输入的字符串保存到了这个数组中。然后,我们遍历整个数组,查找字符 `'i'` 最后一次出现的位置。最后输出结果。需要注意的是,如果数组中没有出现字符 `'i'`,我们需要另外处理这种情况。
相关推荐
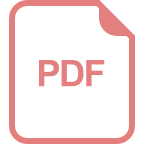
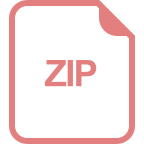
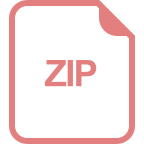













