c++ arx开发,提供一个表格绘制实现类,支持表格合并
时间: 2024-01-28 11:03:46 浏览: 90
好的,以下是一个基于C++的表格绘制实现类,支持表格合并:
```cpp
#include <iostream>
#include <vector>
using namespace std;
// 定义表格中的单元格
struct Cell {
int row;
int col;
int rowSpan;
int colSpan;
string content;
};
// 定义表格类
class Table {
public:
Table(int rows, int cols) {
this->rows = rows;
this->cols = cols;
// 初始化表格
for (int i = 0; i < rows; i++) {
vector<Cell> row;
for (int j = 0; j < cols; j++) {
Cell cell = {i, j, 1, 1, ""};
row.push_back(cell);
}
cells.push_back(row);
}
}
// 设置单元格内容
void setContent(int row, int col, string content) {
cells[row][col].content = content;
}
// 合并单元格
void mergeCells(int row, int col, int rowSpan, int colSpan) {
cells[row][col].rowSpan = rowSpan;
cells[row][col].colSpan = colSpan;
// 清空被合并的单元格
for (int i = row; i < row + rowSpan; i++) {
for (int j = col; j < col + colSpan; j++) {
if (i != row || j != col) {
cells[i][j].row = -1;
cells[i][j].col = -1;
}
}
}
}
// 绘制表格
void drawTable() {
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
Cell cell = cells[i][j];
if (cell.row == -1 && cell.col == -1) {
// 被合并的单元格不输出
continue;
}
if (cell.row == i && cell.col == j) {
// 表格左上角的单元格输出
cout << cell.content << "\t";
}
}
cout << endl;
}
}
private:
int rows;
int cols;
vector<vector<Cell>> cells; // 存储表格中的单元格
};
int main() {
Table table(3, 3);
table.setContent(0, 0, "A");
table.setContent(0, 1, "B");
table.setContent(0, 2, "C");
table.setContent(1, 0, "D");
table.setContent(1, 1, "E");
table.mergeCells(1, 1, 2, 2);
table.setContent(2, 2, "F");
table.drawTable();
return 0;
}
```
在这个表格实现类中,我们使用一个二维数组存储表格中的单元格,每个单元格都有行、列、行跨度、列跨度和内容等属性。在绘制表格时,我们通过遍历单元格数组,判断每个单元格是否需要输出内容,并输出表格。
阅读全文
相关推荐






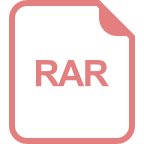

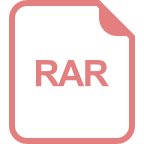


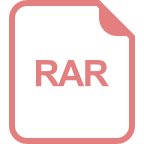
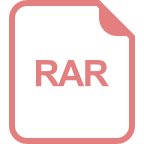





