c++实现电话薄管理程序,Mytel类描述单个电话号码,TelManager类负责管理电话号码。把增、删、改、查功能封装到该类中。要求电话号码能从磁盘读写
时间: 2024-05-07 14:22:59 浏览: 165
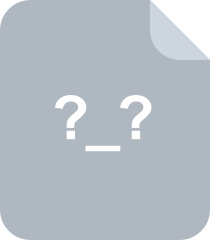
c++电话簿数据系统,用类实现,文件读写,数据段增、删、查、显示
以下是c++实现电话薄管理程序的代码,包括Mytel类和TelManager类:
```c++
#include <iostream>
#include <fstream>
#include <vector>
#include <string>
using namespace std;
class Mytel {
private:
string name;
string phone;
public:
Mytel(string n = "", string p = "") : name(n), phone(p) {}
string getName() { return name; }
string getPhone() { return phone; }
void setName(string n) { name = n; }
void setPhone(string p) { phone = p; }
};
class TelManager {
private:
vector<Mytel> mytels;
public:
void readFromFile(string filename) {
ifstream fin(filename);
if (!fin) {
cout << "File not found!" << endl;
return;
}
mytels.clear();
string name, phone;
while (fin >> name >> phone) {
mytels.push_back(Mytel(name, phone));
}
fin.close();
}
void writeToFile(string filename) {
ofstream fout(filename);
if (!fout) {
cout << "File not found!" << endl;
return;
}
for (int i = 0; i < mytels.size(); i++) {
fout << mytels[i].getName() << " " << mytels[i].getPhone() << endl;
}
fout.close();
}
void add(Mytel mytel) {
mytels.push_back(mytel);
}
void remove(int index) {
if (index >= 0 && index < mytels.size()) {
mytels.erase(mytels.begin()+index);
}
}
void update(int index, Mytel mytel) {
if (index >= 0 && index < mytels.size()) {
mytels[index] = mytel;
}
}
void search(string keyword) {
for (int i = 0; i < mytels.size(); i++) {
if (mytels[i].getName().find(keyword) != string::npos || mytels[i].getPhone().find(keyword) != string::npos) {
cout << mytels[i].getName() << " " << mytels[i].getPhone() << endl;
}
}
}
};
int main() {
TelManager telManager;
telManager.readFromFile("mytels.txt");
while (true) {
cout << "1. Add a phone number" << endl;
cout << "2. Remove a phone number" << endl;
cout << "3. Update a phone number" << endl;
cout << "4. Search phone numbers" << endl;
cout << "5. Exit" << endl;
int choice;
cin >> choice;
if (choice == 1) {
string name, phone;
cout << "Enter name: ";
cin >> name;
cout << "Enter phone: ";
cin >> phone;
telManager.add(Mytel(name, phone));
telManager.writeToFile("mytels.txt");
} else if (choice == 2) {
int index;
cout << "Enter index: ";
cin >> index;
telManager.remove(index);
telManager.writeToFile("mytels.txt");
} else if (choice == 3) {
int index;
string name, phone;
cout << "Enter index: ";
cin >> index;
cout << "Enter name: ";
cin >> name;
cout << "Enter phone: ";
cin >> phone;
telManager.update(index, Mytel(name, phone));
telManager.writeToFile("mytels.txt");
} else if (choice == 4) {
string keyword;
cout << "Enter keyword: ";
cin >> keyword;
telManager.search(keyword);
} else if (choice == 5) {
break;
} else {
cout << "Invalid choice!" << endl;
}
}
return 0;
}
```
在上面的代码中,Mytel类描述单个电话号码,包括名字和电话号码两个属性。TelManager类负责管理电话号码,包括从磁盘读写电话号码、增加、删除、修改、查询电话号码等操作。电话号码可以从磁盘读写,以便程序下次运行时能够恢复之前的电话号码。
在程序运行时,用户可以选择增加、删除、修改、查询电话号码等操作,对电话号码进行管理。每次操作后,程序将电话号码写回磁盘文件中,以便下次运行时能够恢复之前的电话号码。
阅读全文
相关推荐





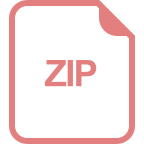
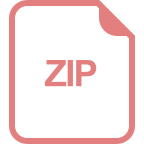
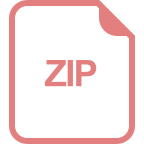
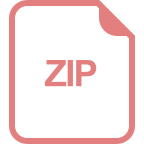
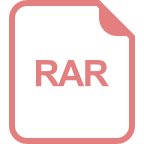