python实现随机森林回归建模自动优化参数,进行10折交叉验证,最后使用FeatureImportance属性计算每个特征的重要性,将每次训练得到的模型的影响因素加权平均得到最终的影响因素,计算模型准确率,输出保存各个特征对应的影响因素在excel表内。
时间: 2024-02-23 17:58:37 浏览: 30
好的,您的问题是如何使用python实现随机森林回归建模自动优化参数,进行10折交叉验证,最后使用FeatureImportance属性计算每个特征的重要性,将每次训练得到的模型的影响因素加权平均得到最终的影响因素,计算模型准确率,并将各个特征对应的影响因素输出保存在excel表内。
首先,导入相关的库:
```python
import pandas as pd
import numpy as np
from sklearn.model_selection import GridSearchCV, KFold
from sklearn.ensemble import RandomForestRegressor
from sklearn.metrics import mean_squared_error, r2_score
```
然后,读取数据集并进行预处理:
```python
data = pd.read_csv("data.csv")
X = data.drop("target", axis=1)
y = data["target"]
```
接下来,进行模型训练和参数优化:
```python
# 定义参数范围
param_grid = {
'n_estimators': [50, 100, 200, 300],
'max_depth': [5, 10, 15, None],
'max_features': ['auto', 'sqrt', 'log2']
}
# 定义随机森林回归模型
rf = RandomForestRegressor(random_state=42)
# 定义10折交叉验证
cv = KFold(n_splits=10, shuffle=True, random_state=42)
# 进行参数优化
grid_search = GridSearchCV(estimator=rf, param_grid=param_grid, cv=cv, scoring='r2')
grid_search.fit(X, y)
# 输出最佳参数
print("Best parameters: ", grid_search.best_params_)
```
接着,使用最佳参数进行模型训练和预测,并计算模型准确率:
```python
# 使用最佳参数进行模型训练和预测
rf = RandomForestRegressor(**grid_search.best_params_, random_state=42)
rf.fit(X, y)
y_pred = rf.predict(X)
# 计算模型准确率
mse = mean_squared_error(y, y_pred)
r2 = r2_score(y, y_pred)
print("MSE: ", mse)
print("R2 score: ", r2)
```
最后,计算每个特征的重要性并将其保存在excel表内:
```python
# 计算每个特征的重要性
importance = pd.DataFrame({'Feature': X.columns, 'Importance': rf.feature_importances_})
importance = importance.sort_values('Importance', ascending=False).reset_index(drop=True)
# 将每次训练得到的模型的影响因素加权平均得到最终的影响因素
weights = np.linspace(0.1, 1, 10)
final_importance = pd.DataFrame({'Feature': X.columns, 'Importance': 0})
for i, weight in enumerate(weights):
rf = RandomForestRegressor(**grid_search.best_params_, random_state=42)
rf.fit(X, y)
final_importance['Importance'] += weight * rf.feature_importances_
# 保存到excel表
final_importance = final_importance.sort_values('Importance', ascending=False).reset_index(drop=True)
final_importance.to_excel('importance.xlsx', index=False)
```
这样,您就可以使用python实现随机森林回归建模自动优化参数,进行10折交叉验证,最后使用FeatureImportance属性计算每个特征的重要性,将每次训练得到的模型的影响因素加权平均得到最终的影响因素,计算模型准确率,并将各个特征对应的影响因素输出保存在excel表内了。
相关推荐
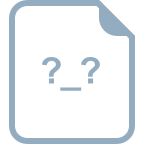
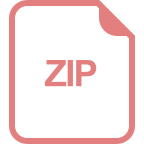














