react native hooks的详细介绍及用法
时间: 2023-11-27 08:49:29 浏览: 80
React Native Hooks 是 React Native 0.59 版本引入的新特性,它可以让我们在不编写类组件的情况下使用状态和其他 React 特性。使用 Hooks 可以让我们更加方便地编写可复用且易于维护的代码。
下面是一些 React Native Hooks 的详细介绍及用法:
1. useState
useState 是 React 中最基本的 Hook 之一,它可以让我们在函数组件中使用状态。useState 接受一个初始值参数,并返回一个数组,其中第一个元素是当前状态值,第二个元素是更新状态值的函数。
例如:
```javascript
import React, { useState } from 'react';
import { Text, View } from 'react-native';
const Example = () => {
const [count, setCount] = useState(0);
return (
<View>
<Text>You clicked {count} times</Text>
<Button onPress={() => setCount(count + 1)} title="Click me" />
</View>
);
};
```
2. useEffect
useEffect 是另一个常用的 Hook,它可以让我们在函数组件中处理副作用。useEffect 接受一个回调函数作为参数,在组件挂载或更新时都会执行这个回调函数。
例如:
```javascript
import React, { useState, useEffect } from 'react';
import { Text, View } from 'react-native';
const Example = () => {
const [count, setCount] = useState(0);
useEffect(() => {
document.title = `You clicked ${count} times`;
});
return (
<View>
<Text>You clicked {count} times</Text>
<Button onPress={() => setCount(count + 1)} title="Click me" />
</View>
);
};
```
3. useContext
useContext 可以让我们在函数组件中使用 React 的上下文。useContext 接受一个上下文对象作为参数,并返回该上下文对象的当前值。
例如:
```javascript
import React, { useContext } from 'react';
import { Text, View } from 'react-native';
const ThemeContext = React.createContext('light');
const Example = () => {
const theme = useContext(ThemeContext);
return (
<View style={{ backgroundColor: theme === 'light' ? '#fff' : '#000' }}>
<Text style={{ color: theme === 'light' ? '#000' : '#fff' }}>
Hello, world!
</Text>
</View>
);
};
```
4. useReducer
useReducer 是 useState 的一种替代方案,它可以让我们更加灵活地管理状态。useReducer 接受一个 reducer 函数和一个初始状态作为参数,并返回一个数组,其中第一个元素是当前状态值,第二个元素是发送 action 的 dispatch 函数。
例如:
```javascript
import React, { useReducer } from 'react';
import { Text, View } from 'react-native';
const initialState = { count: 0 };
function reducer(state, action) {
switch (action.type) {
case 'increment':
return { count: state.count + 1 };
case 'decrement':
return { count: state.count - 1 };
default:
throw new Error();
}
}
const Example = () => {
const [state, dispatch] = useReducer(reducer, initialState);
return (
<View>
<Text>You clicked {state.count} times</Text>
<Button onPress={() => dispatch({ type: 'increment' })} title="+" />
<Button onPress={() => dispatch({ type: 'decrement' })} title="-" />
</View>
);
};
```
以上就是 React Native Hooks 的一些常用用法。需要注意的是,Hooks 只能在函数组件中使用,不能在类组件中使用。同时,不要在循环、条件语句或嵌套函数中使用 Hooks,以免出现意外的行为。
阅读全文
相关推荐
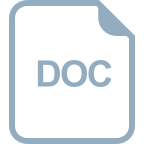
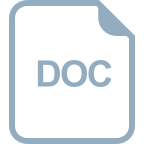
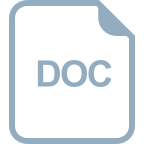
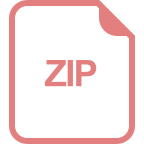
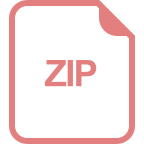
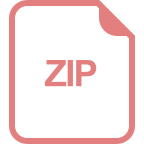
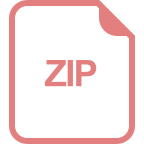
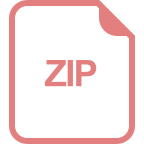
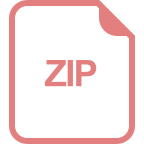
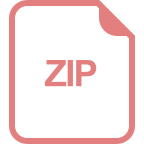
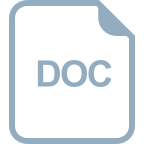
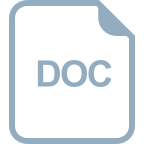
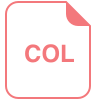

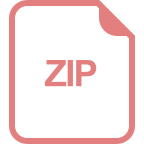
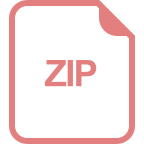
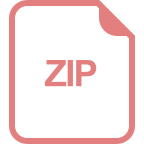
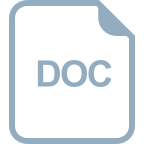
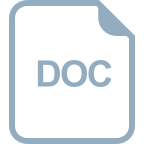