用java和MySQL实现访客管理系统的黑名单增删改查功能,并且已经包含了异常处理的代码,
时间: 2023-06-17 15:08:55 浏览: 92
以下是一个简单的访客管理系统的黑名单增删改查功能的Java代码,使用MySQL作为数据库:
```java
import java.sql.*;
public class VisitorManagementSystem {
private static final String DB_URL = "jdbc:mysql://localhost:3306/visitor_management";
private static final String DB_USER = "root";
private static final String DB_PASSWORD = "password";
private Connection conn = null;
public VisitorManagementSystem() throws SQLException {
try {
Class.forName("com.mysql.jdbc.Driver");
conn = DriverManager.getConnection(DB_URL, DB_USER, DB_PASSWORD);
} catch (ClassNotFoundException e) {
System.out.println("MySQL JDBC driver not found.");
e.printStackTrace();
}
}
public void addVisitorToBlacklist(String name) {
try {
PreparedStatement stmt = conn.prepareStatement("INSERT INTO blacklist (name) VALUES (?)");
stmt.setString(1, name);
stmt.executeUpdate();
System.out.println(name + " has been added to the blacklist.");
} catch (SQLException e) {
System.out.println("Failed to add " + name + " to the blacklist.");
e.printStackTrace();
}
}
public void removeVisitorFromBlacklist(String name) {
try {
PreparedStatement stmt = conn.prepareStatement("DELETE FROM blacklist WHERE name = ?");
stmt.setString(1, name);
int rowsUpdated = stmt.executeUpdate();
if (rowsUpdated > 0) {
System.out.println(name + " has been removed from the blacklist.");
} else {
System.out.println(name + " is not in the blacklist.");
}
} catch (SQLException e) {
System.out.println("Failed to remove " + name + " from the blacklist.");
e.printStackTrace();
}
}
public void updateVisitorInBlacklist(String oldName, String newName) {
try {
PreparedStatement stmt = conn.prepareStatement("UPDATE blacklist SET name = ? WHERE name = ?");
stmt.setString(1, newName);
stmt.setString(2, oldName);
int rowsUpdated = stmt.executeUpdate();
if (rowsUpdated > 0) {
System.out.println(oldName + " has been updated to " + newName + " in the blacklist.");
} else {
System.out.println(oldName + " is not in the blacklist.");
}
} catch (SQLException e) {
System.out.println("Failed to update " + oldName + " in the blacklist.");
e.printStackTrace();
}
}
public void searchVisitorInBlacklist(String name) {
try {
PreparedStatement stmt = conn.prepareStatement("SELECT name FROM blacklist WHERE name = ?");
stmt.setString(1, name);
ResultSet rs = stmt.executeQuery();
if (rs.next()) {
System.out.println(name + " is in the blacklist.");
} else {
System.out.println(name + " is not in the blacklist.");
}
} catch (SQLException e) {
System.out.println("Failed to search " + name + " in the blacklist.");
e.printStackTrace();
}
}
public void closeConnection() throws SQLException {
if (conn != null) {
conn.close();
}
}
public static void main(String[] args) {
try {
VisitorManagementSystem vms = new VisitorManagementSystem();
vms.addVisitorToBlacklist("John");
vms.addVisitorToBlacklist("Mary");
vms.searchVisitorInBlacklist("John");
vms.searchVisitorInBlacklist("Jane");
vms.updateVisitorInBlacklist("John", "Johnny");
vms.searchVisitorInBlacklist("John");
vms.searchVisitorInBlacklist("Johnny");
vms.removeVisitorFromBlacklist("Mary");
vms.removeVisitorFromBlacklist("Jane");
vms.closeConnection();
} catch (SQLException e) {
System.out.println("Failed to connect to the database.");
e.printStackTrace();
}
}
}
```
上述代码中,我们首先定义了数据库的相关参数,然后在构造函数中使用JDBC驱动程序连接到MySQL数据库。我们实现了四个功能,分别为添加黑名单、删除黑名单、更新黑名单和搜索黑名单。对于每个功能,我们使用PreparedStatement对象执行SQL语句,使用try-catch块进行异常处理。
在main函数中,我们创建了一个VisitorManagementSystem对象,并演示了上述四个功能。最后,我们关闭了数据库连接。
阅读全文
相关推荐
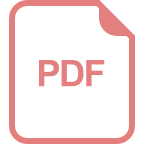








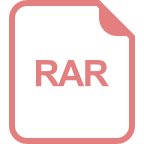
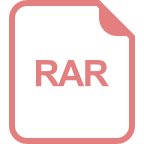
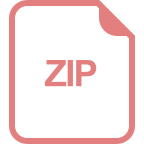
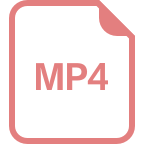