友元函数的加号运算符重载
时间: 2023-11-19 14:06:39 浏览: 91
友元函数的加号运算符重载通常用于实现两个对象相加的操作。在类中定义加号运算符重载函数时,必须将该函数声明为友元函数,以便该函数能够直接访问该类的私有成员变量。友元函数的声明方式为:`friend 返回类型 operator+(const 类名& obj1, const 类名& obj2);`
其中,`obj1`和`obj2`代表两个要相加的对象,`返回类型`代表相加后的结果类型。在函数中,可以使用两个对象的私有成员变量进行计算,并返回计算结果。例如:
```
class MyClass {
private:
int x;
public:
MyClass(int val) : x(val) {}
friend MyClass operator+(const MyClass& obj1, const MyClass& obj2);
};
MyClass operator+(const MyClass& obj1, const MyClass& obj2) {
return MyClass(obj1.x + obj2.x);
}
```
在上述代码中,我们定义了一个名为`MyClass`的类,并在其中定义了一个名为`operator+`的友元函数,该函数用于将两个`MyClass`类型的对象相加。在函数中,我们使用两个对象的私有成员变量`x`进行计算,最终返回一个新的`MyClass`对象,该对象的`x`值为相加后的结果。
相关问题
有两个矩阵a 和b ,均为2 行3 列。求两个矩阵之和。用成员函数重载运算符" + " ,使之能用于矩阵相加,如c=a+b。用友元函数重载运算符" - " ,使之能用于矩阵相减,如d=a-b。并以矩阵的形式输出c和d的值。
可以这样实现:
```c++
#include <iostream>
using namespace std;
class Matrix {
private:
int data[2][3];
public:
Matrix() {} // 默认构造函数
Matrix(int d[2][3]) { // 带参数的构造函数
for (int i = 0; i < 2; i++) {
for (int j = 0; j < 3; j++) {
data[i][j] = d[i][j];
}
}
}
Matrix operator+(const Matrix& matrix) { // 重载加号运算符
int d[2][3];
for (int i = 0; i < 2; i++) {
for (int j = 0; j < 3; j++) {
d[i][j] = data[i][j] + matrix.data[i][j];
}
}
return Matrix(d);
}
friend Matrix operator-(const Matrix& m1, const Matrix& m2); // 声明友元函数
void print() { // 输出矩阵
for (int i = 0; i < 2; i++) {
for (int j = 0; j < 3; j++) {
cout << data[i][j] << " ";
}
cout << endl;
}
}
};
Matrix operator-(const Matrix& m1, const Matrix& m2) { // 实现友元函数
int d[2][3];
for (int i = 0; i < 2; i++) {
for (int j = 0; j < 3; j++) {
d[i][j] = m1.data[i][j] - m2.data[i][j];
}
}
return Matrix(d);
}
int main() {
int a[2][3] = { {1, 2, 3}, {4, 5, 6} };
int b[2][3] = { {7, 8, 9}, {10, 11, 12} };
Matrix m1(a), m2(b);
cout << "a + b = " << endl;
Matrix m3 = m1 + m2;
m3.print();
cout << "a - b = " << endl;
Matrix m4 = m1 - m2;
m4.print();
return 0;
}
```
输出结果为:
```
a + b =
8 10 12
14 16 18
a - b =
-6 -6 -6
-6 -6 -6
```
如何在C++中通过友元函数实现自定义类型加号运算符的重载?请提供一个具体的示例代码。
在C++中,运算符重载是一个强大的特性,它允许我们对自定义数据类型定义运算符的操作。特别是当运算符重载为友元函数时,它可以提供更加灵活的操作方式。为了更好地理解这个概念并掌握其实用技巧,建议查阅《C++运算符重载友元函数详解:从基础到实战》。这本书详细介绍了运算符重载的机制和友元函数的概念,以及如何在实际项目中应用它们。
参考资源链接:[C++运算符重载友元函数详解:从基础到实战](https://wenku.csdn.net/doc/842hct228r?spm=1055.2569.3001.10343)
具体到加号运算符的重载,它涉及到定义一个新的操作符函数,使其能够处理自定义数据类型之间的加法。在下面的示例中,我们将展示如何为一个简单的自定义复数类(Complex)实现加号运算符的重载:
```cpp
#include <iostream>
// 自定义复数类
class Complex {
private:
double real;
double imag;
public:
// 构造函数
Complex(double r = 0.0, double i = 0.0) : real(r), imag(i) {}
// 友元函数声明
friend Complex operator+(const Complex&, const Complex&);
};
// 加号运算符的重载实现,作为友元函数
Complex operator+(const Complex& c1, const Complex& c2) {
return Complex(c1.real + c2.real, c1.imag + c2.imag);
}
// 输出复数的函数
std::ostream& operator<<(std::ostream& out, const Complex& c) {
out << c.real <<
参考资源链接:[C++运算符重载友元函数详解:从基础到实战](https://wenku.csdn.net/doc/842hct228r?spm=1055.2569.3001.10343)
阅读全文
相关推荐
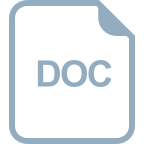



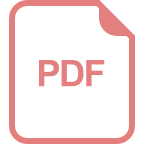
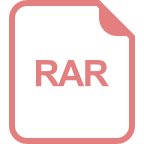
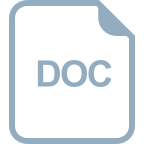
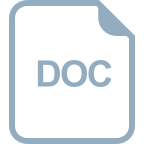





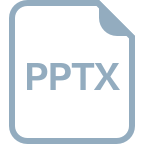