用java实现线性表
时间: 2023-09-27 20:05:52 浏览: 54
线性表是一种常见的数据结构,可以使用Java语言来实现。下面是一个简单的线性表实现示例:
```java
public class MyArrayList<E> {
private int size; // 线性表的大小
private Object[] elementData; // 存储元素的数组
// 构造函数
public MyArrayList() {
this.size = 0;
this.elementData = new Object[10]; // 初始化数组大小为10
}
// 获取线性表的大小
public int size() {
return this.size;
}
// 判断线性表是否为空
public boolean isEmpty() {
return this.size == 0;
}
// 获取指定位置的元素
public E get(int index) {
if (index < 0 || index >= size) {
throw new IndexOutOfBoundsException("Index out of range: " + index);
}
return (E) elementData[index];
}
// 添加元素到线性表尾部
public void add(E e) {
ensureCapacity(size + 1); // 确保容量足够
elementData[size++] = e; // 将元素添加到数组中
}
// 在指定位置插入元素
public void add(int index, E e) {
if (index < 0 || index > size) {
throw new IndexOutOfBoundsException("Index out of range: " + index);
}
ensureCapacity(size + 1); // 确保容量足够
System.arraycopy(elementData, index, elementData, index + 1, size - index); // 将元素后移
elementData[index] = e; // 插入新元素
size++; // 更新线性表大小
}
// 删除指定位置的元素
public E remove(int index) {
if (index < 0 || index >= size) {
throw new IndexOutOfBoundsException("Index out of range: " + index);
}
E oldValue = (E) elementData[index]; // 获取要删除的元素
int numMoved = size - index - 1;
if (numMoved > 0) {
System.arraycopy(elementData, index + 1, elementData, index, numMoved); // 将元素前移
}
elementData[--size] = null; // 将最后一个元素置空
return oldValue;
}
// 确保数组容量足够
private void ensureCapacity(int minCapacity) {
int oldCapacity = elementData.length;
if (minCapacity > oldCapacity) {
int newCapacity = oldCapacity * 2;
if (newCapacity < minCapacity) {
newCapacity = minCapacity;
}
elementData = Arrays.copyOf(elementData, newCapacity); // 扩容数组
}
}
}
```
在上面的示例中,我们使用一个Object类型的数组来存储元素,并通过泛型来支持不同类型的元素。通过实现常用的方法,我们可以对线性表进行添加、删除、获取等操作。需要注意的是,当线性表的大小超过数组容量时,我们需要动态扩容数组,以保证能够继续添加元素。
相关推荐
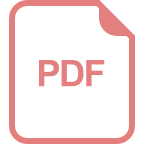













