数据库课程设计mysql python代码
时间: 2023-08-07 16:55:56 浏览: 52
以下是一个简单的示例,展示如何使用Python连接MySQL数据库,并执行一些基本的操作:
```python
import mysql.connector
# 连接数据库
cnx = mysql.connector.connect(user='your_username', password='your_password',
host='your_host', database='your_database')
# 创建游标
cursor = cnx.cursor()
# 创建表
create_table_query = """
CREATE TABLE students (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(50),
age INT,
major VARCHAR(50)
)
"""
cursor.execute(create_table_query)
# 插入数据
insert_data_query = """
INSERT INTO students (name, age, major)
VALUES ('John', 20, 'Computer Science'),
('Jane', 22, 'Mathematics'),
('Mike', 21, 'Physics')
"""
cursor.execute(insert_data_query)
# 查询数据
select_data_query = "SELECT * FROM students"
cursor.execute(select_data_query)
result = cursor.fetchall()
for row in result:
print(row)
# 更新数据
update_data_query = "UPDATE students SET major = 'Chemistry' WHERE id = 2"
cursor.execute(update_data_query)
# 删除数据
delete_data_query = "DELETE FROM students WHERE id = 3"
cursor.execute(delete_data_query)
# 提交更改
cnx.commit()
# 关闭游标和连接
cursor.close()
cnx.close()
```
请注意,你需要将代码中的`your_username`、`your_password`、`your_host`和`your_database`替换为你自己的MySQL数据库的信息。
以上代码演示了如何创建表格,插入数据,查询数据,更新数据和删除数据等基本操作。你可以根据自己的需求进行扩展和修改。
相关推荐
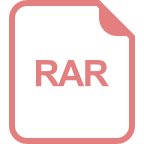
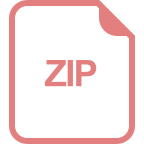














