创建一个Dog类,向其中添加eat()方法。使用AOP在eat()方法的前后输出任意一段文字(使用注解方式实现)
时间: 2024-05-07 18:17:20 浏览: 91
好的,我可以帮您回答这个问题。
首先,我们需要创建一个Dog类,并在其中添加eat()方法。代码如下:
```java
public class Dog {
public void eat() {
System.out.println("The dog is eating...");
}
}
```
接下来,我们需要使用AOP来在eat()方法的前后输出任意一段文字。这可以使用注解方式实现。
首先,我们需要在pom.xml文件中添加以下依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-aop</artifactId>
</dependency>
```
然后,我们可以创建一个注解,用于标记需要进行AOP处理的方法。代码如下:
```java
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.METHOD)
public @interface LogExecutionTime {
}
```
在注解中,我们使用@Retention和@Target两个元注解,分别表示该注解在运行时可见,并且只能应用在方法上。
接下来,我们需要创建一个Aspect类,用于实现AOP的逻辑。代码如下:
```java
@Component
@Aspect
public class LoggingAspect {
private final Logger logger = LoggerFactory.getLogger(this.getClass());
@Around("@annotation(LogExecutionTime)")
public Object logExecutionTime(ProceedingJoinPoint joinPoint) throws Throwable {
long start = System.currentTimeMillis();
logger.info("Method {}() start.", joinPoint.getSignature().getName());
Object proceed = joinPoint.proceed();
logger.info("Method {}() end, execution time: {}ms.", joinPoint.getSignature().getName(), System.currentTimeMillis() - start);
return proceed;
}
}
```
在Aspect类中,我们使用@Around注解,表示需要在注解所标记的方法前后进行处理。在注解中,我们使用@annotation指定了需要处理的注解类型为LogExecutionTime。
在logExecutionTime方法中,我们首先记录了方法的开始执行时间,并输出了一条日志。然后,我们通过调用joinPoint.proceed()方法执行了注解所标记的方法。最后,我们再次记录了用时,并输出了一条日志。
最后,我们就可以在Dog类的eat()方法上加上LogExecutionTime注解,来启用AOP处理了。代码如下:
```java
public class Dog {
@LogExecutionTime
public void eat() {
System.out.println("The dog is eating...");
}
}
```
这样,当我们调用Dog类的eat()方法时,就会在方法执行前后输出一条日志了。
希望这个回答能够帮到您,如果您有任何其他问题或需求,欢迎随时向我提问。
阅读全文
相关推荐





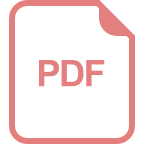

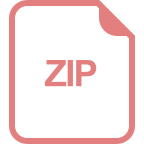
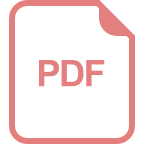
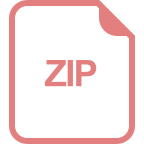
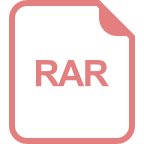
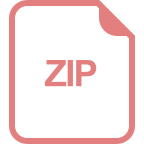
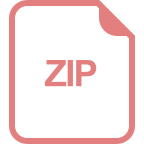
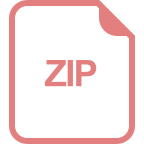