利用C++实现:电影管理:实现电影信息和用户信息维护的基本操作。如 电影的增加、修改、删除、查找、显示等。其中,电影信息包括电影编号、名称、评分、类型、国别、主演、时长等信 息
时间: 2023-09-18 08:09:27 浏览: 36
下面是一个简单的电影管理系统的实现示例,包括电影信息和用户信息的基本操作:
```cpp
#include <iostream>
#include <vector>
#include <algorithm>
class Movie {
private:
int id; // 电影编号
std::string title; // 电影名称
float rating; // 评分
std::string genre; // 类型
std::string country; // 国别
std::string actor; // 主演
int duration; // 时长(分钟)
public:
// 构造函数
Movie(int id, const std::string& title, float rating, const std::string& genre,
const std::string& country, const std::string& actor, int duration)
: id(id), title(title), rating(rating), genre(genre),
country(country), actor(actor), duration(duration) {}
// 获取电影编号
int getId() const {
return id;
}
// 获取电影名称
std::string getTitle() const {
return title;
}
// 获取评分
float getRating() const {
return rating;
}
// 获取类型
std::string getGenre() const {
return genre;
}
// 获取国别
std::string getCountry() const {
return country;
}
// 获取主演
std::string getActor() const {
return actor;
}
// 获取时长
int getDuration() const {
return duration;
}
};
class MovieManager {
private:
std::vector<Movie> movies; // 电影信息存储
public:
// 添加电影
void addMovie(const Movie& movie) {
movies.push_back(movie);
}
// 修改电影
void updateMovie(int id, const Movie& updatedMovie) {
for (auto& movie : movies) {
if (movie.getId() == id) {
movie = updatedMovie;
break;
}
}
}
// 删除电影
void deleteMovie(int id) {
movies.erase(std::remove_if(movies.begin(), movies.end(),
[id](const Movie& movie) { return movie.getId() == id; }),
movies.end());
}
// 查找电影
Movie* findMovie(int id) {
for (auto& movie : movies) {
if (movie.getId() == id) {
return &movie;
}
}
return nullptr;
}
// 显示所有电影
void displayMovies() const {
for (const auto& movie : movies) {
std::cout << "ID: " << movie.getId() << std::endl;
std::cout << "Title: " << movie.getTitle() << std::endl;
std::cout << "Rating: " << movie.getRating() << std::endl;
std::cout << "Genre: " << movie.getGenre() << std::endl;
std::cout << "Country: " << movie.getCountry() << std::endl;
std::cout << "Actor: " << movie.getActor() << std::endl;
std::cout << "Duration: " << movie.getDuration() << " minutes" << std::endl;
std::cout << "---------------------------------" << std::endl;
}
}
};
int main() {
// 创建电影管理器
MovieManager manager;
// 添加电影
manager.addMovie(Movie(1, "Movie 1", 8.5, "Action", "USA", "Actor 1", 120));
manager.addMovie(Movie(2, "Movie 2", 7.9, "Drama", "UK", "Actor 2", 150));
manager.addMovie(Movie(3, "Movie 3", 6.8, "Comedy", "China", "Actor 3", 110));
// 显示所有电影
manager.displayMovies();
// 修改电影
manager.updateMovie(2, Movie(2, "Updated Movie 2", 8.0, "Drama", "UK", "Actor 4", 160));
// 显示所有电影
manager.displayMovies();
// 删除电影
manager.deleteMovie(1);
// 显示所有电影
manager.displayMovies();
// 查找电影
Movie* foundMovie = manager.findMovie(3);
if (foundMovie) {
std::cout << "Found Movie: " << foundMovie->getTitle() << std::endl;
} else {
std::cout << "Movie not found!" << std::endl;
}
return 0;
}
```
以上示例实现了电影管理系统的基本功能,包括添加电影、修改电影、删除电影、查找电影和显示所有电影的操作。你可以根据需要进行适当的修改和扩展。请注意,在实际的应用中可能需要更复杂的数据结构和操作,这里只是提供了一个基本的框架供参考。
相关推荐
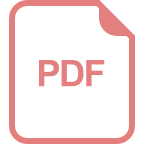
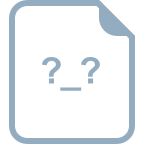
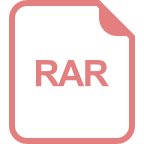














