android studio 用代码写出图书管理界面,该界面中底部有4个导航栏,分别是首页、场馆、书架和我 的,分别对应4个不同的Fragment的布局样式
时间: 2024-02-01 11:14:03 浏览: 28
好的,让我们来写一个简单的图书管理界面,代码如下:
1. MainActivity.java
```java
public class MainActivity extends AppCompatActivity {
private BottomNavigationView bottomNavigationView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
bottomNavigationView = findViewById(R.id.bottom_navigation);
// 设置默认选中的导航栏
bottomNavigationView.setSelectedItemId(R.id.navigation_home);
// 监听导航栏的切换事件
bottomNavigationView.setOnNavigationItemSelectedListener(new BottomNavigationView.OnNavigationItemSelectedListener() {
@Override
public boolean onNavigationItemSelected(@NonNull MenuItem item) {
Fragment fragment = null;
switch (item.getItemId()) {
case R.id.navigation_home:
fragment = new HomeFragment();
break;
case R.id.navigation_venue:
fragment = new VenueFragment();
break;
case R.id.navigation_bookshelf:
fragment = new BookshelfFragment();
break;
case R.id.navigation_me:
fragment = new MeFragment();
break;
}
// 替换当前的Fragment
getSupportFragmentManager().beginTransaction().replace(R.id.fragment_container, fragment).commit();
return true;
}
});
}
}
```
2. activity_main.xml
```xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:id="@+id/layout_root"
android:layout_width="match_parent"
android:layout_height="match_parent">
<FrameLayout
android:id="@+id/fragment_container"
android:layout_width="0dp"
android:layout_height="0dp"
app:layout_constraintBottom_toTopOf="@id/bottom_navigation"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<com.google.android.material.bottomnavigation.BottomNavigationView
android:id="@+id/bottom_navigation"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:background="@color/white"
app:itemIconTint="@drawable/bottom_navigation_item_color"
app:itemTextColor="@drawable/bottom_navigation_item_color"
app:labelVisibilityMode="labeled"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:menu="@menu/bottom_navigation_menu" />
</androidx.constraintlayout.widget.ConstraintLayout>
```
3. bottom_navigation_menu.xml
```xml
<menu xmlns:android="http://schemas.android.com/apk/res/android">
<item
android:id="@+id/navigation_home"
android:icon="@drawable/ic_home"
android:title="@string/navigation_home" />
<item
android:id="@+id/navigation_venue"
android:icon="@drawable/ic_venue"
android:title="@string/navigation_venue" />
<item
android:id="@+id/navigation_bookshelf"
android:icon="@drawable/ic_bookshelf"
android:title="@string/navigation_bookshelf" />
<item
android:id="@+id/navigation_me"
android:icon="@drawable/ic_me"
android:title="@string/navigation_me" />
</menu>
```
4. HomeFragment.java
```java
public class HomeFragment extends Fragment {
public HomeFragment() {
// Required empty public constructor
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// Inflate the layout for this fragment
return inflater.inflate(R.layout.fragment_home, container, false);
}
}
```
5. fragment_home.xml
```xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:text="Home Fragment"
android:textSize="30sp"
android:layout_gravity="center"/>
</LinearLayout>
```
6. VenueFragment.java
```java
public class VenueFragment extends Fragment {
public VenueFragment() {
// Required empty public constructor
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// Inflate the layout for this fragment
return inflater.inflate(R.layout.fragment_venue, container, false);
}
}
```
7. fragment_venue.xml
```xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:text="Venue Fragment"
android:textSize="30sp"
android:layout_gravity="center"/>
</LinearLayout>
```
8. BookshelfFragment.java
```java
public class BookshelfFragment extends Fragment {
public BookshelfFragment() {
// Required empty public constructor
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// Inflate the layout for this fragment
return inflater.inflate(R.layout.fragment_bookshelf, container, false);
}
}
```
9. fragment_bookshelf.xml
```xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:text="Bookshelf Fragment"
android:textSize="30sp"
android:layout_gravity="center"/>
</LinearLayout>
```
10. MeFragment.java
```java
public class MeFragment extends Fragment {
public MeFragment() {
// Required empty public constructor
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// Inflate the layout for this fragment
return inflater.inflate(R.layout.fragment_me, container, false);
}
}
```
11. fragment_me.xml
```xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:text="Me Fragment"
android:textSize="30sp"
android:layout_gravity="center"/>
</LinearLayout>
```
以上就是一个简单的图书管理界面,包含4个不同的Fragment的布局样式和底部的导航栏。
相关推荐
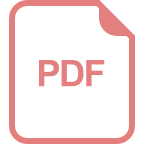
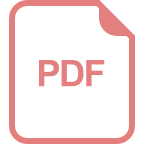














