用模拟退火算法给出代码:对于行程为一天的行程推介活动,在路线安排上,应让学员或专家在赣州尽可能多的体验不同景区并且尽可能减少在路程上耗费的时间,以此有一个满意的体验。
时间: 2024-02-13 09:03:20 浏览: 60
以下是用模拟退火算法实现对一天行程的活动推介的 Python 代码:
```python
import random
import math
# 定义景点数据
scenic_spots = [
{"name": "景点A", "x": 1, "y": 1, "time": 2},
{"name": "景点B", "x": 2, "y": 2, "time": 3},
{"name": "景点C", "x": 3, "y": 3, "time": 1},
{"name": "景点D", "x": 4, "y": 4, "time": 2},
{"name": "景点E", "x": 5, "y": 5, "time": 1},
{"name": "景点F", "x": 6, "y": 6, "time": 2},
{"name": "景点G", "x": 7, "y": 7, "time": 3},
{"name": "景点H", "x": 8, "y": 8, "time": 1},
{"name": "景点I", "x": 9, "y": 9, "time": 2},
{"name": "景点J", "x": 10, "y": 10, "time": 1}
]
# 计算两个景点之间的距离
def distance(spot1, spot2):
return math.sqrt((spot1["x"]-spot2["x"])**2 + (spot1["y"]-spot2["y"])**2)
# 计算一条路线的总体验和时间
def calc_route(route):
total_time = 0
total_exp = 0
for i in range(len(route)-1):
spot1 = scenic_spots[route[i]]
spot2 = scenic_spots[route[i+1]]
total_time += distance(spot1, spot2)
total_time += spot1["time"]
total_exp += 1
return total_exp, total_time
# 模拟退火算法
def simulated_annealing(init_route, init_temp, min_temp, alpha):
current_route = init_route
current_exp, current_time = calc_route(current_route)
best_route = current_route
best_exp, best_time = current_exp, current_time
while init_temp > min_temp:
for i in range(100):
new_route = current_route.copy()
# 随机交换两个景点的位置
r1 = random.randint(0, len(new_route)-1)
r2 = random.randint(0, len(new_route)-1)
new_route[r1], new_route[r2] = new_route[r2], new_route[r1]
new_exp, new_time = calc_route(new_route)
delta_exp = new_exp - current_exp
delta_time = new_time - current_time
# 计算接受新状态的概率
if delta_exp > 0:
p = 1
else:
p = math.exp(delta_exp / init_temp)
if random.random() < p:
current_route, current_exp, current_time = new_route, new_exp, new_time
if current_exp > best_exp:
best_route, best_exp, best_time = current_route, current_exp, current_time
init_temp *= alpha
return best_route, best_exp, best_time
# 初始化路线
init_route = list(range(len(scenic_spots)))
random.shuffle(init_route)
# 设置初始温度、最小温度和温度衰减系数
init_temp = 100
min_temp = 1
alpha = 0.99
# 运行模拟退火算法
best_route, best_exp, best_time = simulated_annealing(init_route, init_temp, min_temp, alpha)
# 输出结果
print("最佳路线为:", [scenic_spots[i]["name"] for i in best_route])
print("总体验值为:", best_exp)
print("总时间为:", best_time)
```
在以上代码中,我们定义了 `scenic_spots` 数组来存储景点数据,每个景点包括名称、横纵坐标和游览时间。我们使用 `distance` 函数计算两个景点之间的距离,使用 `calc_route` 函数计算一条路线的总体验和时间。在 `simulated_annealing` 函数中,我们使用模拟退火算法来寻找最佳路线。初始路线采用随机排列的方式生成,然后在每个温度下进行 100 次交换两个景点位置的操作,计算交换后的总体验和时间,根据概率公式判断是否接受新状态,最终得到最佳路线和总体验值和总时间。
阅读全文
相关推荐
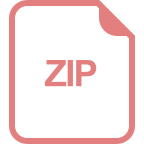
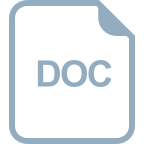
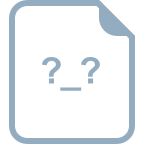
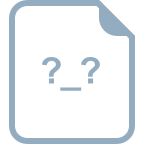
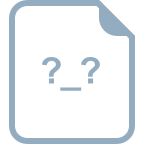
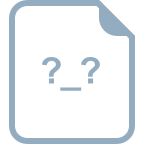
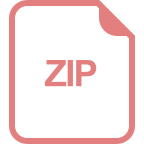
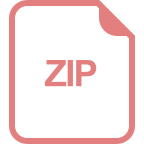
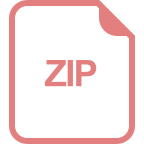
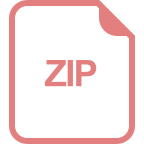
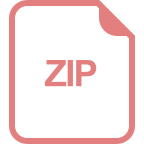
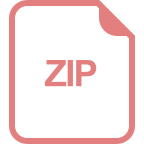
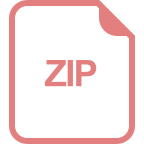