redis如何给mysql做缓存
时间: 2023-07-20 17:34:35 浏览: 89
Redis可以作为MySQL的缓存,主要有两种方式:
1. 将MySQL查询结果缓存在Redis中,下次查询时先从Redis中读取,如果Redis中没有,则从MySQL中读取,并将结果缓存到Redis中。这种方式需要在应用程序代码中实现,可以使用Jedis或Lettuce等Java客户端库来连接Redis,同时需要使用MySQL提供的官方驱动或第三方库来连接MySQL。下面是一个简单的Java代码示例:
```java
import redis.clients.jedis.Jedis;
import java.sql.*;
public class Main {
public static void main(String[] args) {
String redisHost = "localhost";
int redisPort = 6379;
String mysqlUrl = "jdbc:mysql://localhost:3306/mydatabase";
String mysqlUser = "root";
String mysqlPassword = "mypassword";
String query = "SELECT * FROM mytable WHERE id = ?";
int id = 123;
try {
// Connect to Redis
Jedis jedis = new Jedis(redisHost, redisPort);
// Check if the result is already in Redis
String result = jedis.get("query:" + query + ":" + id);
if (result == null) {
// Connect to MySQL
Connection connection = DriverManager.getConnection(mysqlUrl, mysqlUser, mysqlPassword);
PreparedStatement statement = connection.prepareStatement(query);
statement.setInt(1, id);
ResultSet resultSet = statement.executeQuery();
// Read the result from MySQL
if (resultSet.next()) {
result = resultSet.getString("result");
// Cache the result in Redis
jedis.set("query:" + query + ":" + id, result);
}
resultSet.close();
statement.close();
connection.close();
}
System.out.println("Result: " + result);
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
2. 通过MySQL的插件或代理将查询转发到Redis,如果Redis中没有缓存,则继续转发到MySQL,并将结果缓存到Redis中。这种方式不需要修改应用程序代码,但需要安装和配置相应的MySQL插件或代理,如ProxySQL、MySQL-Redis-Proxy等。这种方式可以实现自动缓存,但需要额外的配置和管理工作。
需要注意的是,使用Redis作为MySQL的缓存可以提高查询性能,但也会增加系统复杂度和管理成本,需要根据具体情况进行选择。
阅读全文
相关推荐


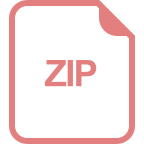
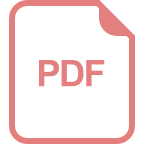
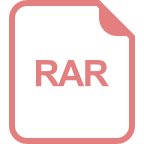
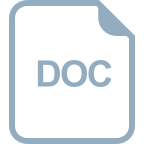









