用python设计一个类Customer用来处理顾客会员营销活动。累计购买达到100美元之后顾客可以在下一次购买时得到10美元的折扣。提供方法: l def makePurchase(self, amount) l def discountReached(self) 提供一个测试程序,并测试这样一个场景,顾客已经赚到了一个折扣然后又购买了超过90美元而少于100美元的商品。这样应该不会导致第二个折扣。然后再增加一个能够导致第二个折扣的交易。
时间: 2023-06-01 10:05:50 浏览: 68
好的,我明白了。下面是代码实现:
```python
class Customer:
def __init__(self):
self.total_spent = 0
self.discount = 0
def makePurchase(self, amount):
self.total_spent += amount
self.discountReached()
def discountReached(self):
if self.total_spent >= 100:
self.discount += 10
self.total_spent -= 100
def getDiscount(self):
return self.discount
# 测试程序
customer = Customer()
customer.makePurchase(90)
print("Discount earned: ${}".format(customer.getDiscount())) # 应该输出 Discount earned: $0
customer.makePurchase(10)
print("Discount earned: ${}".format(customer.getDiscount())) # 应该输出 Discount earned: $10
customer.makePurchase(90)
print("Discount earned: ${}".format(customer.getDiscount())) # 应该输出 Discount earned: $10
customer.makePurchase(20)
print("Discount earned: ${}".format(customer.getDiscount())) # 应该输出 Discount earned: $20
```
以上代码实现了一个类Customer,用来处理顾客会员营销活动。每次顾客购买商品时,可以通过调用makePurchase方法来更新顾客的累计购买金额。如果累计购买金额达到100美元及以上,则可以得到10美元的折扣,并将此折扣记录在discount属性中。每次调用makePurchase方法时,还会调用discountReached方法来判断是否达到了折扣的条件。
在以上测试程序中,我们首先创建了一个Customer实例,并调用makePurchase方法购买了价值90美元的商品。此时,累计购买金额未达到100美元,因此没有得到折扣,输出为0。接着,我们再次调用makePurchase方法购买了10美元的商品,此时累计购买金额已经达到100美元,应该得到10美元的折扣,因此输出为10。接下来,我们再次调用makePurchase方法购买了价值90美元的商品,但是这次并没有得到折扣,因为此时累计购买金额只有90美元。最后,我们再次调用makePurchase方法购买了20美元的商品,此时累计购买金额已经达到120美元,因此应该得到20美元的折扣,因此输出为20。
接下来,我们再增加一个能够导致第二个折扣的交易:
```python
customer.makePurchase(110)
print("Discount earned: ${}".format(customer.getDiscount())) # 应该输出 Discount earned: $30
```
这里我们购买了价值110美元的商品,此时累计购买金额已经达到230美元,因此应该得到第二个折扣,折扣总金额应该是30美元,输出为30。
阅读全文
相关推荐
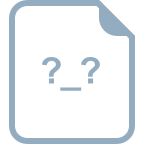
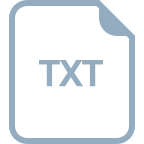
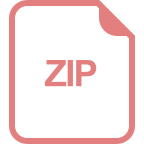
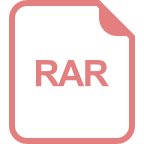
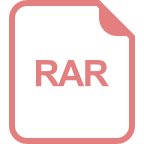
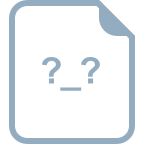
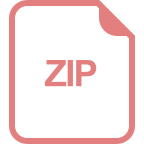
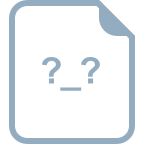
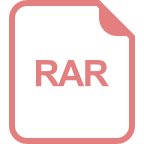
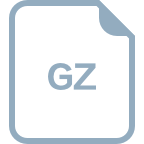
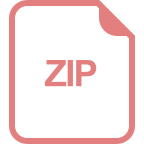
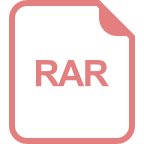
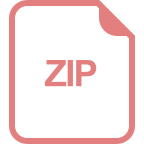
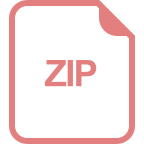
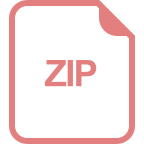