封装react-native监听手机系统自带的按钮并自己可以设置需要返回的页面的组件
时间: 2024-04-06 18:34:53 浏览: 25
您可以通过封装一个高阶组件(Higher Order Component, HOC)来实现监听手机系统自带的按钮并自己设置需要返回的页面的组件。以下是一个简单的实现:
```
import React from 'react';
import { BackHandler } from 'react-native';
const withCustomBackHandler = (WrappedComponent, onBackPress) => {
class WithCustomBackHandler extends React.Component {
constructor(props) {
super(props);
this.handleBackPress = this.handleBackPress.bind(this);
}
componentDidMount() {
BackHandler.addEventListener('hardwareBackPress', this.handleBackPress);
}
componentWillUnmount() {
BackHandler.removeEventListener('hardwareBackPress', this.handleBackPress);
}
handleBackPress() {
const { navigation } = this.props;
if (onBackPress && onBackPress()) {
// 如果onBackPress返回了true,则表示已经处理了后退事件
return true;
} else if (navigation && navigation.goBack) {
// 如果没有处理后退事件,则执行默认的后退操作
navigation.goBack();
return true;
}
return false;
}
render() {
return <WrappedComponent {...this.props} />;
}
}
return WithCustomBackHandler;
};
export default withCustomBackHandler;
```
这个高阶组件接受两个参数:WrappedComponent和onBackPress。WrappedComponent是需要封装的组件,onBackPress是一个可选的回调函数,用于在用户按下后退按钮时执行一些自定义的操作。
在组件挂载时,我们使用BackHandler.addEventListener方法来添加一个事件监听器,以便在用户按下后退按钮时调用handleBackPress方法。
在handleBackPress方法中,我们首先尝试调用onBackPress方法,如果它返回了true,则表示已经处理了后退事件,我们不需要执行默认的后退操作。否则,我们检查组件的navigation对象是否存在并且有goBack方法,如果有,则执行默认的后退操作。
最后,我们返回一个新的组件WithCustomBackHandler,它包装了WrappedComponent,并传递了所有的props。当用户按下后退按钮时,WithCustomBackHandler会截获该事件,并根据onBackPress的返回值执行相应的操作。
相关推荐
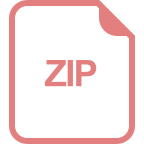
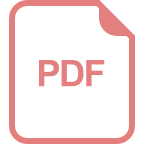











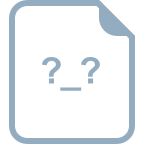