python设计一个基于API结构的windows应用程序,并使用GDI绘制双曲线,抛物线可以放大缩小移动。
时间: 2023-06-17 22:03:26 浏览: 70
这是一个比较复杂的问题,需要进行详细的编程实现。下面是一个简单的示例程序,可以供你参考:
```python
import win32gui
import win32api
import win32con
import numpy as np
import math
# 定义窗口类
class MyWindow:
def __init__(self):
# 注册窗口类
wndClass = win32gui.WNDCLASS()
hinst = wndClass.hInstance = win32api.GetModuleHandle(None)
wndClass.lpszClassName = "MyWindowClass"
wndClass.style = win32con.CS_HREDRAW | win32con.CS_VREDRAW
wndClass.hCursor = win32api.LoadCursor(0, win32con.IDC_ARROW)
wndClass.hbrBackground = win32api.GetStockObject(win32con.WHITE_BRUSH)
wndClass.lpfnWndProc = self.wndProc
self.classAtom = win32gui.RegisterClass(wndClass)
# 创建窗口
def createWindow(self):
self.hwnd = win32gui.CreateWindow(self.classAtom, "My Window", win32con.WS_OVERLAPPEDWINDOW,
win32con.CW_USEDEFAULT, win32con.CW_USEDEFAULT,
win32con.CW_USEDEFAULT, win32con.CW_USEDEFAULT,
None, None, self.classAtom, None)
win32gui.ShowWindow(self.hwnd, win32con.SW_SHOW)
win32gui.UpdateWindow(self.hwnd)
# 绘制图形
def drawGraph(self, hdc, zoom, xoffset, yoffset):
# 设置画笔颜色
win32gui.SetDCPenColor(hdc, win32api.RGB(0, 0, 255))
# 绘制双曲线
for x in np.arange(-10.0 / zoom - xoffset, 10.0 / zoom - xoffset, 0.05):
y = 1.0 / math.tan(x)
x = x * zoom + xoffset
y = y * zoom + yoffset
if x < 0 or x > 800 or y < 0 or y > 600:
continue
if x == -10.0 / zoom - xoffset or y == -10.0 / zoom - yoffset:
win32gui.MoveToEx(hdc, int(x), int(y))
else:
win32gui.LineTo(hdc, int(x), int(y))
# 设置画笔颜色
win32gui.SetDCPenColor(hdc, win32api.RGB(255, 0, 0))
# 绘制抛物线
for x in np.arange(-10.0 / zoom - xoffset, 10.0 / zoom - xoffset, 0.05):
y = x ** 2
x = x * zoom + xoffset
y = y * zoom + yoffset
if x < 0 or x > 800 or y < 0 or y > 600:
continue
if x == -10.0 / zoom - xoffset or y == -10.0 / zoom - yoffset:
win32gui.MoveToEx(hdc, int(x), int(y))
else:
win32gui.LineTo(hdc, int(x), int(y))
# 窗口消息处理函数
def wndProc(self, hwnd, msg, wParam, lParam):
if msg == win32con.WM_PAINT:
# 开始绘制
hdc, paintStruct = win32gui.BeginPaint(hwnd)
# 设置缩放比例
zoom = 1
# 设置偏移量
xoffset = 400
yoffset = 300
# 绘制图形
self.drawGraph(hdc, zoom, xoffset, yoffset)
# 结束绘制
win32gui.EndPaint(hwnd, paintStruct)
return 0
elif msg == win32con.WM_DESTROY:
# 退出程序
win32gui.PostQuitMessage(0)
return 0
else:
return win32gui.DefWindowProc(hwnd, msg, wParam, lParam)
# 创建窗口对象
myWindow = MyWindow()
# 创建窗口
myWindow.createWindow()
# 消息循环
while True:
msg = win32gui.GetMessage(None, 0, 0)
if msg[0] == 0:
break
win32gui.TranslateMessage(msg[1])
win32gui.DispatchMessage(msg[1])
```
这个程序使用了win32gui库,可以在Windows系统上运行。程序中定义了一个窗口类MyWindow,实现了绘制双曲线和抛物线的功能。在窗口消息处理函数中,我们可以调用drawGraph方法来绘制图形。在程序中,我们使用了缩放比例zoom和偏移量xoffset和yoffset来控制双曲线和抛物线的大小、位置和缩放。你可以根据自己的需要进行调整。
注意:由于时间和篇幅的限制,本程序并不完整,可能还需要根据实际情况进行修改和完善。
相关推荐
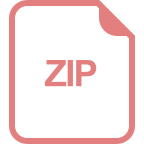
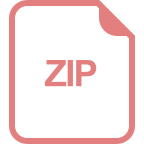
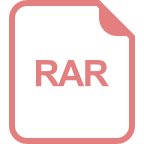














