如何发送HttpPost发送的表单格式,在另一个程序接收
时间: 2024-09-08 19:03:31 浏览: 110
要在C#中发送一个HttpPost请求,你可以使用`HttpClient`类。以下是一个示例代码,展示了如何发送包含表单数据的HttpPost请求:
```csharp
using System;
using System.Net.Http;
using System.Threading.Tasks;
using System.Collections.Generic;
class Program
{
static async Task Main(string[] args)
{
using (var client = new HttpClient())
{
// 设置请求的URL
var requestUri = "http://example.com/api/endpoint";
// 创建表单内容
var formContent = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("key1", "value1"),
new KeyValuePair<string, string>("key2", "value2"),
// 更多的键值对
});
// 发送HttpPost请求
try
{
var response = await client.PostAsync(requestUri, formContent);
response.EnsureSuccessStatusCode(); // 确保我们得到了成功响应
var responseBody = await response.Content.ReadAsStringAsync();
Console.WriteLine(responseBody);
}
catch(HttpRequestException e)
{
// 处理异常
Console.WriteLine("\nException Caught!");
Console.WriteLine("Message :{0} ",e.Message);
}
}
}
}
```
在另一个程序中接收HttpPost表单数据,如果是Web应用程序,你通常会使用ASP.NET或ASP.NET Core框架。以下是ASP.NET Core控制器接收表单数据的示例代码:
```csharp
using Microsoft.AspNetCore.Mvc;
[ApiController]
[Route("[controller]")]
public class FormController : ControllerBase
{
[HttpPost]
public IActionResult ReceiveForm([FromForm] Dictionary<string, string> formData)
{
// 处理接收到的表单数据
foreach (var entry in formData)
{
Console.WriteLine($"{entry.Key}: {entry.Value}");
}
return Ok();
}
}
```
在这个例子中,`[FromForm]`属性用于自动绑定请求体中的表单数据到`formData`参数上。
阅读全文
相关推荐
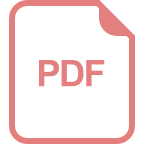
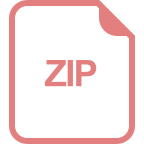
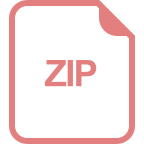
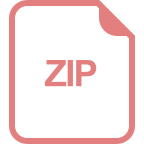
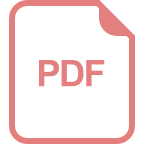
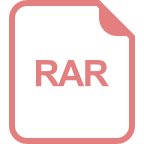
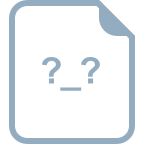
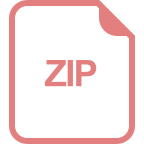
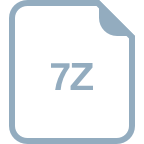
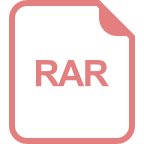
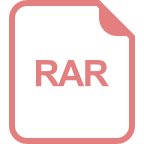
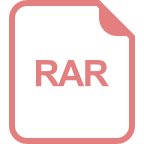
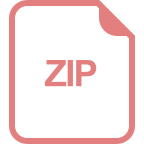
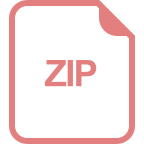
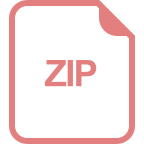
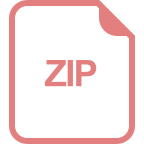
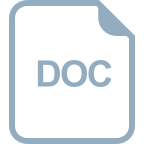
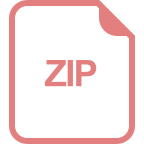