vue3中实现一个父页面的弹窗功能,描述显隐和传参的实现逻辑
时间: 2024-09-26 19:04:09 浏览: 13
在Vue3中,实现一个父页面的弹窗功能通常涉及到组件的父子通信以及状态管理。以下是简单的实现逻辑:
1. **创建组件**:
- 创建一个独立的弹窗组件,比如`Modal.vue`,它将包含显示和关闭的逻辑。
```vue
<template>
<div class="modal" v-if="isShowing">
<!-- 弹窗内容 -->
<slot></slot>
<button @click="close">关闭</button>
</div>
</template>
<script>
export default {
props: {
title: {
type: String,
required: true,
},
content: {
type: String,
required: true,
},
onClose: {
type: Function,
default: () => {},
},
},
data() {
return {
isShowing: false,
};
},
methods: {
show() {
this.isShowing = true;
},
close() {
this.isShowing = false;
this.onClose();
},
},
};
</script>
```
2. **父组件引用**:
- 在父组件(例如`ParentComponent.vue`)里引入弹窗组件,并传递所需参数。
```vue
<template>
<button @click="showModal('标题', '内容')">打开弹窗</button>
<Modal v-model="modalIsShowing" :title="modalTitle" :content="modalContent" @on-close="handleClose"></Modal>
</template>
<script>
import Modal from './Modal.vue';
export default {
components: {
Modal,
},
data() {
return {
modalTitle: '',
modalContent: '',
modalIsShowing: false,
};
},
methods: {
showModal(title, content) {
this.modalTitle = title;
this.modalContent = content;
this.modalIsShowing = true;
},
handleClose() {
this.modalIsShowing = false; // 关闭弹窗
},
},
};
</script>
```
3. **控制显示与隐藏**:
- 父组件通过`v-model`绑定`modalIsShowing`来控制弹窗是否显示,点击按钮调用`showModal`方法开启弹窗,关闭则触发`handleClose`方法。
4. **传参**:
- 通过`props`属性将标题和内容传递给弹窗组件,当需要更改内容时,只需修改相应的属性即可。
相关推荐
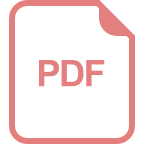
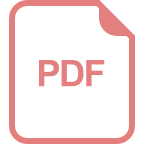
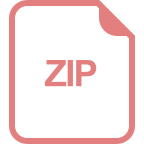














