如何在Unity中使用C#从头开始实现语音通话功能?
时间: 2024-12-21 14:23:03 浏览: 2
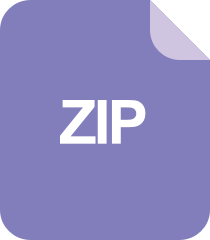
Json 技术实现登入注册
在Unity中使用C#实现语音通话功能通常需要借助一些第三方库,如Microsoft的Azure Cognitive Services Speech SDK或开源的AISpeech。以下是基本步骤:
1. **安装插件**:
- 如果选择Azure Speech,你需要在Unity Package Manager中搜索并安装`Microsoft.CognitiveServices.Speech`插件。
- 对于AISpeech等其他库,也需下载对应版本并在项目中引用。
2. **设置认证**:
- 在Azure Speech服务中创建一个资源,并获取访问Key和SAS Token,用于身份验证。
3. **初始化语音识别/合成引擎**:
```csharp
using Microsoft.CognitiveServices.Speech;
var speechConfig = SpeechConfig.FromSubscription("你的订阅密钥", "你的服务区域");
speechConfig.SpeechRecognitionLanguage = "en-US"; // 使用的语言代码
```
4. **语音识别** (接收语音输入):
```csharp
using UnityEngine.UI;
public class VoiceInput : MonoBehaviour
{
private SpeechRecognizer recognizer;
void Start()
{
recognizer = new SpeechRecognizer(speechConfig);
recognizer.RecognizeOnceAsync(Recognized += OnSpeechRecognized);
}
private void OnSpeechRecognized(SpeechRecognitionResult result)
{
if (result.Reason == ResultReason.RecognizedSpeech)
Debug.Log($"你说的是: {result.Text}");
}
}
```
5. **语音合成** (发送文本转语音):
```csharp
public class TextToSpeech : MonoBehaviour
{
private SpeechSynthesizer synthesizer;
void Start()
{
synthesizer = new SpeechSynthesizer();
synthesizer.SetOutputToDefaultAudioDevice();
synthesizer.SpeakTextAsync("你好,这是语音合成的例子");
}
}
```
6. **连接网络**:
实时语音通话可能需要网络支持,你可以通过WebSocket或其他实时通信协议建立连接。
记得在实际应用中处理错误、异常以及网络连接等问题。以上是一个基础框架,具体的实现可能会因为使用的库的不同而有所差异。
阅读全文
相关推荐
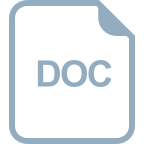
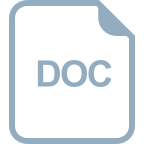
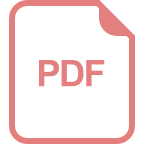
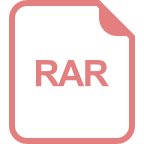
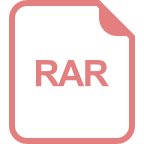
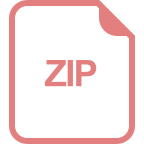
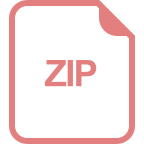
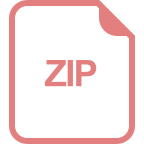
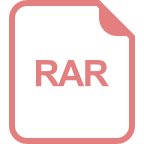
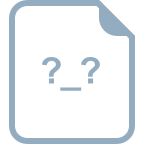
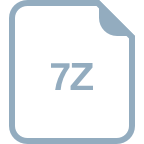
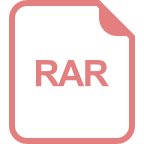
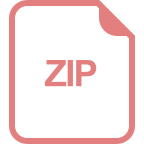
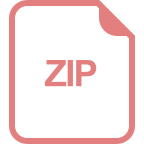