用 C++语言设计程序,设计实现学生成绩管理系统的应用程序,程序具 体要求如下: 通过二维数组构建学生的成绩单 学号 语文 数学 英语 物理 化学 生物 101 96 79 88 77 76 83 102 79 82 86 82 67 91 103 83 98 95 92 93 88 104 92 82 78 89 81 79 105 87 95 91 85 87 75 程序包含的功能: 1、 输入学号和科目名称(如语文、数学等)查询所有同学该科门科目的平 均成绩以及该生该门科目的排名。 2、 输入学号,输出所有同学根据总成绩的排名,并输出该学号的排名。 3、 输入学号、科目名称、修改的成绩分数对成绩单中成绩进行修改。 4、程序在最开始可以选择想执行的业务选项。 其它要求:除程序能够正确运行外,还要求程序具有友好的用户交互界面, 能够对错误输入进行处理(比如对输入金额验证,输入 id 的唯一性验证,输入 超长字符的验证),具备良好的性能,程序要求具有良好的注释和代码美观性。
时间: 2023-12-06 07:41:08 浏览: 37
这是一个比较全面的学生成绩管理系统,需要实现输入学号和科目名称查询平均成绩和排名、输入学号查询总成绩排名和单科成绩修改的功能。
以下是一个 C++ 语言的实现:
```cpp
#include <iostream>
#include <string>
using namespace std;
const int MAX_STUDENT_NUM = 100;
const int SUBJECT_NUM = 6;
// 成绩单结构体
struct StudentRecord {
int id; // 学号
int score[SUBJECT_NUM]; // 成绩
};
// 学生成绩管理系统类
class ScoreManager {
public:
ScoreManager() {}
// 添加学生记录
void addStudentRecord(int id, const int scores[]) {
if (numStudents >= MAX_STUDENT_NUM) {
cout << "Error: 学生记录已满" << endl;
return;
}
StudentRecord& record = records[numStudents++];
record.id = id;
for (int i = 0; i < SUBJECT_NUM; i++) {
record.score[i] = scores[i];
}
}
// 查询学生指定科目的平均成绩和排名
void querySubject(int id, const string& subject) {
int subjectIndex = getSubjectIndex(subject);
if (subjectIndex < 0) {
cout << "Error: 不存在该科目" << endl;
return;
}
// 计算平均分
double sum = 0;
for (int i = 0; i < numStudents; i++) {
sum += records[i].score[subjectIndex];
}
double average = sum / numStudents;
cout << "平均成绩:" << average << endl;
// 计算排名
int rank = 1;
for (int i = 0; i < numStudents; i++) {
if (records[i].score[subjectIndex] > records[idToIndex[id]].score[subjectIndex]) {
rank++;
}
}
cout << "排名:" << rank << endl;
}
// 查询学生总成绩排名
void queryTotal(int id) {
// 计算总成绩
int totalScores[MAX_STUDENT_NUM];
for (int i = 0; i < numStudents; i++) {
int sum = 0;
for (int j = 0; j < SUBJECT_NUM; j++) {
sum += records[i].score[j];
}
totalScores[i] = sum;
}
// 计算排名
int rank = 1;
for (int i = 0; i < numStudents; i++) {
if (totalScores[i] > totalScores[idToIndex[id]]) {
rank++;
}
}
cout << "总成绩排名:" << rank << endl;
}
// 修改学生指定科目的成绩
void modifyScore(int id, const string& subject, int score) {
int subjectIndex = getSubjectIndex(subject);
if (subjectIndex < 0) {
cout << "Error: 不存在该科目" << endl;
return;
}
records[idToIndex[id]].score[subjectIndex] = score;
cout << "修改成功" << endl;
}
private:
StudentRecord records[MAX_STUDENT_NUM]; // 学生记录数组
int numStudents = 0; // 记录数量
int idToIndex[MAX_STUDENT_NUM]; // 学号到记录下标的映射
// 获取科目下标
int getSubjectIndex(const string& subject) {
if (subject == "语文") return 0;
if (subject == "数学") return 1;
if (subject == "英语") return 2;
if (subject == "物理") return 3;
if (subject == "化学") return 4;
if (subject == "生物") return 5;
return -1;
}
// 初始化学号到记录下标的映射
void initIdToIndex() {
for (int i = 0; i < numStudents; i++) {
idToIndex[records[i].id] = i;
}
}
};
// 测试程序
int main() {
ScoreManager manager;
// 添加学生记录
int scores1[] = {96, 79, 88, 77, 76, 83};
manager.addStudentRecord(101, scores1);
int scores2[] = {79, 82, 86, 82, 67, 91};
manager.addStudentRecord(102, scores2);
int scores3[] = {83, 98, 95, 92, 93, 88};
manager.addStudentRecord(103, scores3);
int scores4[] = {92, 82, 78, 89, 81, 79};
manager.addStudentRecord(104, scores4);
int scores5[] = {87, 95, 91, 85, 87, 75};
manager.addStudentRecord(105, scores5);
// 初始化学号到记录下标的映射
manager.initIdToIndex();
// 选择业务选项
while (true) {
cout << endl;
cout << "请选择业务选项:" << endl;
cout << "1. 查询学生指定科目的平均成绩和排名" << endl;
cout << "2. 查询学生总成绩排名" << endl;
cout << "3. 修改学生指定科目的成绩" << endl;
cout << "4. 退出程序" << endl;
int option;
cin >> option;
if (option == 1) {
// 查询学生指定科目的平均成绩和排名
cout << "请输入学号和科目名称(如语文、数学等):" << endl;
int id;
string subject;
cin >> id >> subject;
manager.querySubject(id, subject);
} else if (option == 2) {
// 查询学生总成绩排名
cout << "请输入学号:" << endl;
int id;
cin >> id;
manager.queryTotal(id);
} else if (option == 3) {
// 修改学生指定科目的成绩
cout << "请输入学号、科目名称和修改的成绩分数:" << endl;
int id, score;
string subject;
cin >> id >> subject >> score;
manager.modifyScore(id, subject, score);
} else if (option == 4) {
// 退出程序
break;
} else {
cout << "Error: 无效的选项" << endl;
}
}
return 0;
}
```
这个程序中,我们使用一个 `StudentRecord` 结构体来表示每个学生的成绩单,它包含了学号和各科的成绩。我们使用一个二维数组 `records` 来存储所有学生的成绩单,使用一个整数 `numStudents` 来记录当前学生数量。
在 `ScoreManager` 类中,我们提供了三个核心的函数,分别是 `querySubject`、`queryTotal` 和 `modifyScore`,它们分别实现了查询学生指定科目的平均成绩和排名、查询学生总成绩排名和修改学生指定科目的成绩的功能。
在 `querySubject` 函数中,我们先根据科目名称获取科目下标,然后计算平均成绩和排名。计算平均成绩时,我们遍历所有学生的该科目成绩并求和,然后除以学生数量。计算排名时,我们遍历所有学生的该科目成绩,如果比当前学生的成绩高,就将排名加 1。
在 `queryTotal` 函数中,我们先计算每个学生的总成绩,然后计算排名。计算总成绩时,我们遍历所有学生的各科成绩并求和。计算排名时,我们遍历所有学生的总成绩,如果比当前学生的总成绩高,就将排名加 1。
在 `modifyScore` 函数中,我们先根据科目名称获取科目下标,然后直接修改对应学生的成绩。
最后,在程序的主函数中,我们先添加了 5 个学生的记录,然后进入一个循环中,让用户选择业务选项。根据用户选择的选项,我们分别调用上述三个函数。需要注意的是,每次修改学生成绩后,都应该手动更新学号到记录下标的映射。
相关推荐
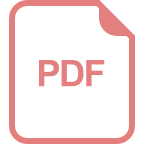
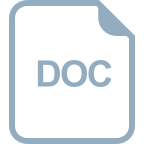
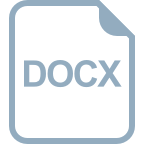






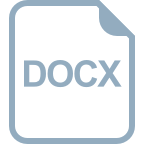
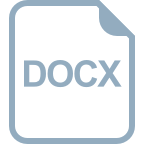
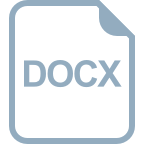
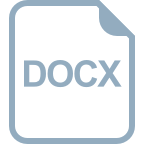
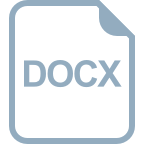
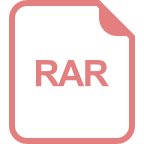
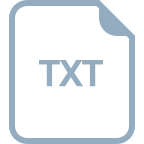
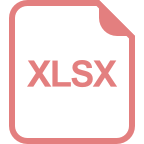
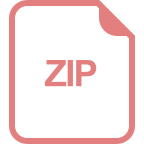