el-input 关闭时清除输入
时间: 2023-10-08 22:04:05 浏览: 43
可以通过监听 el-input 的 blur 事件,在事件回调函数中清空输入框的值。示例代码如下:
```html
<template>
<el-input v-model="inputValue" @blur="handleInputBlur"></el-input>
</template>
<script>
export default {
data() {
return {
inputValue: ''
}
},
methods: {
handleInputBlur() {
this.inputValue = ''
}
}
}
</script>
```
相关问题
el-table嵌套el-input不能输入
el-table嵌套el-input不能输入的问题可能是由于以下原因导致的:
1. el-input组件没有绑定双向响应式数据(v-model)。
2. 组件嵌套太深还是该组件是一个坑(具体原因不清楚,只知道解决方法)。
解决方案如下:
1. 在data中定义一个变量,然后在el-input组件中使用v-model进行双向数据绑定,这样子就会解决el-input组件无法输入的问题了。
2. 将el-input组件放在el-table组件外部,或者使用其他组件代替el-input组件。
以下是两种解决方案的代码示例:
1. 在el-table中使用v-model进行双向数据绑定
```html
<el-table :data="tableData">
<el-table-column prop="name" label="姓名"></el-table-column>
<el-table-column prop="age" label="年龄">
<template slot-scope="scope">
<el-input v-model="scope.row.age"></el-input>
</template>
</el-table-column>
</el-table>
```
2. 将el-input组件放在el-table组件外部
```html
<el-table :data="tableData">
<el-table-column prop="name" label="姓名"></el-table-column>
<el-table-column prop="age" label="年龄">
<template slot-scope="scope">
{{ scope.row.age }}
</template>
</el-table-column>
</el-table>
<el-input v-model="inputValue"></el-input>
```
el-input-number 只能输入正整数
el-input-number 是 Element UI 中的一个组件,用于输入数字的输入框。如果你想限制 el-input-number 只能输入正整数,可以通过设置 min 属性为 1 来实现。这样用户就只能输入大于等于 1 的整数值。
以下是一个示例代码:
```html
<template>
<el-input-number v-model="number" :min="1" :precision="0"></el-input-number>
</template>
<script>
export default {
data() {
return {
number: 1
};
}
};
</script>
```
在上面的代码中,我们将 el-input-number 绑定到了一个名为 number 的数据属性上,并设置了 min 属性为 1,precision 属性为 0。这样用户只能输入大于等于 1 的整数值。
相关推荐
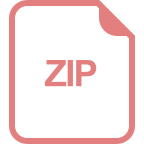
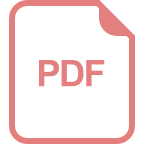












