swift中 UITableViewCell高度是自适应的, cell里面有个多行显示的UILabel,SnapKit下,这个UILabel距离父视图contentView的top和left和right 为0 ,高度为根据字符串自适应。外部传进来一个长度小于等于3图片Array数组,如果数组长度为0或者为空,这个UILabel的高度为cell的高度,距离父视图contentView的bottom为0。如果数组不为空,那就遍历创ImageView,每个ImageView宽高为78,100,,从左向右间距为10这样排列显示, 并在数组里面取image给ImageView。每个ImageView 顶部距离UILabel为10 ,底部距离父视图contentView的bottom为0,怎么写
时间: 2024-03-02 17:47:48 浏览: 114
可以在`UITableViewDelegate`协议的`tableView(_:heightForRowAt:)`方法中根据数据源的不同来计算不同的行高。在此方法中,我们可以使用`systemLayoutSizeFitting(_:withHorizontalFittingPriority:verticalFittingPriority:)`方法来计算自适应高度的UILabel的高度,然后再根据图片数组的情况来计算整个UITableViewCell的高度。
具体实现步骤如下:
1. 在UITableViewCell的`init`方法中创建UILabel和UIImageView,使用SnapKit布局。
```
class CustomTableViewCell: UITableViewCell {
let label = UILabel()
var imageViews = [UIImageView]()
override init(style: UITableViewCell.CellStyle, reuseIdentifier: String?) {
super.init(style: style, reuseIdentifier: reuseIdentifier)
// 创建UILabel
contentView.addSubview(label)
label.snp.makeConstraints { (make) in
make.top.left.right.equalToSuperview()
}
label.numberOfLines = 0
// 创建UIImageView
for _ in 0..<3 {
let imageView = UIImageView()
contentView.addSubview(imageView)
imageView.snp.makeConstraints { (make) in
make.top.equalTo(label.snp.bottom).offset(10)
make.width.equalTo(78)
make.height.equalTo(100)
if let lastImageView = imageViews.last {
make.left.equalTo(lastImageView.snp.right).offset(10)
} else {
make.left.equalToSuperview()
}
}
imageView.contentMode = .scaleAspectFill
imageView.clipsToBounds = true
imageViews.append(imageView)
}
}
}
```
2. 在数据源方法`tableView(_:cellForRowAt:)`中根据数据源设置UILabel和UIImageView的内容,并根据图片数组的情况计算UITableViewCell的高度。
```
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCell(withIdentifier: "CustomTableViewCell", for: indexPath) as! CustomTableViewCell
// 设置UILabel的内容和高度
cell.label.text = "这是一段很长的文本,需要自适应高度。"
let labelSize = cell.label.systemLayoutSizeFitting(CGSize(width: cell.frame.width, height: CGFloat.greatestFiniteMagnitude), withHorizontalFittingPriority: .required, verticalFittingPriority: .fittingSizeLevel)
cell.label.snp.updateConstraints { (make) in
make.height.equalTo(labelSize.height)
}
// 根据图片数组设置UIImageView的内容并计算UITableViewCell的高度
if let images = imagesArray[indexPath.row], !images.isEmpty {
for i in 0..<cell.imageViews.count {
if i < images.count {
cell.imageViews[i].image = images[i]
cell.imageViews[i].isHidden = false
} else {
cell.imageViews[i].isHidden = true
}
}
let imageViewHeight = ceil(Double(images.count) / 3.0) * 100.0 + ceil(Double(images.count - 1) / 3.0) * 10.0
cell.contentView.snp.updateConstraints { (make) in
make.bottom.equalTo(cell.imageViews.last!.snp.bottom).offset(10)
}
return cell
} else {
for imageView in cell.imageViews {
imageView.isHidden = true
}
cell.contentView.snp.updateConstraints { (make) in
make.bottom.equalTo(cell.label.snp.bottom)
}
return cell
}
}
```
3. 在`tableView(_:heightForRowAt:)`方法中根据UILabel和UIImageView的高度计算UITableViewCell的高度。
```
func tableView(_ tableView: UITableView, heightForRowAt indexPath: IndexPath) -> CGFloat {
let label = UILabel()
label.numberOfLines = 0
label.text = "这是一段很长的文本,需要自适应高度。"
let labelSize = label.systemLayoutSizeFitting(CGSize(width: tableView.frame.width, height: CGFloat.greatestFiniteMagnitude), withHorizontalFittingPriority: .required, verticalFittingPriority: .fittingSizeLevel)
if let images = imagesArray[indexPath.row], !images.isEmpty {
let imageViewHeight = ceil(Double(images.count) / 3.0) * 100.0 + ceil(Double(images.count - 1) / 3.0) * 10.0
return labelSize.height + 10.0 + CGFloat(imageViewHeight) + 10.0
} else {
return labelSize.height
}
}
```
相关推荐
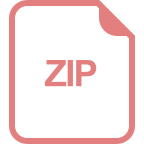
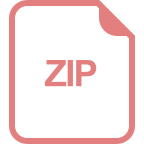
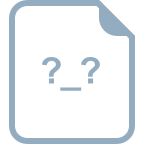









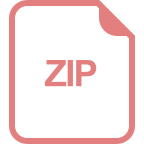
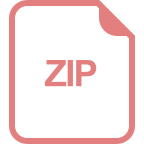
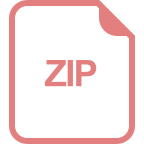
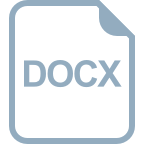
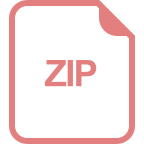
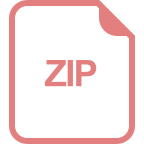
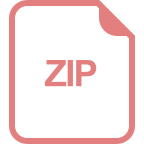
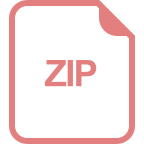