C++输入一个年份一个月份一个日期判断其是否正确
时间: 2024-03-25 10:35:46 浏览: 109
可以使用C++自带的日期库`<chrono>`和`<ctime>`,通过`std::chrono::system_clock::now()`获取当前时间,再通过`std::chrono::system_clock::to_time_t()`将其转换为`time_t`类型,最后用`std::localtime()`将其转换为`tm`类型,即可得到当前时间的年月日。然后再将输入的年月日与当前时间进行比较,判断其是否正确。
以下是示例代码:
```c++
#include <iostream>
#include <chrono>
#include <ctime>
bool is_valid_date(int year, int month, int day) {
// 获取当前时间
std::chrono::system_clock::time_point now = std::chrono::system_clock::now();
std::time_t now_time = std::chrono::system_clock::to_time_t(now);
std::tm* now_tm = std::localtime(&now_time);
// 判断年份是否正确
if (year < 0 || year > now_tm->tm_year + 1900) {
return false;
}
// 判断月份是否正确
if (month < 1 || month > 12) {
return false;
}
// 判断日期是否正确
int days_in_month[12] = {31, 28 + (year % 4 == 0 && (year % 100 != 0 || year % 400 == 0)), 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
if (day < 1 || day > days_in_month[month - 1]) {
return false;
}
return true;
}
int main() {
int year, month, day;
std::cout << "请输入年份、月份、日期:" << std::endl;
std::cin >> year >> month >> day;
if (is_valid_date(year, month, day)) {
std::cout << "输入的日期正确!" << std::endl;
} else {
std::cout << "输入的日期不正确!" << std::endl;
}
return 0;
}
```
该程序会从用户那里读取输入,然后检查输入的年月日是否正确。需要注意的是,该代码仅检查年份、月份和日期是否在合法范围内,而不会考虑一些特殊的日期,比如2月29日这样的闰年日期。如果需要检查这样的日期,需要对代码进行相应的修改。
阅读全文
相关推荐
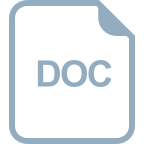
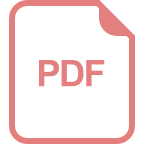
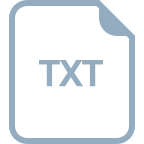
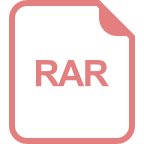
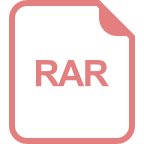
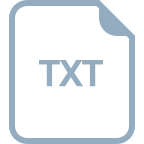
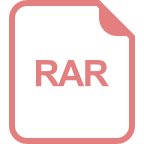
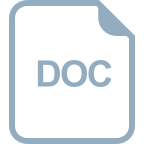
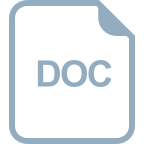
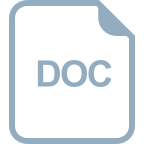
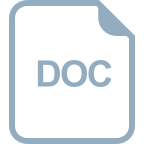
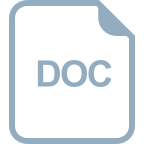
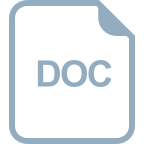
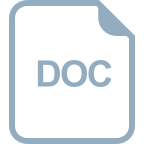




