在Dev-C++软件中用c语言代码实现拥塞控制算法仿真动画
时间: 2023-12-28 08:04:18 浏览: 45
好的,以下是在 Dev-C++ 软件中用 C 语言代码实现拥塞控制算法仿真动画的示例程序。该程序使用了 Win32 API 中的 GDI+ 绘图库来绘制动画界面。
```c
#include <stdio.h>
#include <windows.h>
#include <gdiplus.h>
#pragma comment (lib,"Gdiplus.lib")
#define ID_TIMER 1 // 定时器 ID
#define WIN_WIDTH 800 // 窗口宽度
#define WIN_HEIGHT 600 // 窗口高度
#define RECT_WIDTH 20 // 拥塞窗口矩形宽度
#define RECT_HEIGHT 20 // 拥塞窗口矩形高度
#define RECT_SPACING 5 // 拥塞窗口矩形间距
#define SLOW_START_COLOR RGB(0, 128, 255) // 慢启动阶段矩形颜色
#define CONGESTION_AVOIDANCE_COLOR RGB(255, 128, 0) // 拥塞避免阶段矩形颜色
#define TIMEOUT_COLOR RGB(255, 0, 0) // 超时阶段矩形颜色
int cwnd = 1; // 拥塞窗口大小
int ssthresh = 16; // 慢启动阈值
int rtt = 100; // 往返时延
int state = 0; // 状态:0-慢启动,1-拥塞避免,2-超时
void drawRect(Gdiplus::Graphics& graphics, int x, int y, int width, int height, COLORREF color);
LRESULT CALLBACK WndProc(HWND hwnd, UINT msg, WPARAM wParam, LPARAM lParam)
{
switch (msg)
{
case WM_CREATE:
SetTimer(hwnd, ID_TIMER, rtt, NULL);
break;
case WM_TIMER:
if (state == 2) {
// 发生超时
ssthresh = cwnd / 2;
cwnd = 1;
state = 0;
} else if (cwnd >= ssthresh) {
// 进入拥塞避免状态
state = 1;
cwnd += 1;
} else {
// 进入慢启动状态
state = 0;
cwnd *= 2;
}
InvalidateRect(hwnd, NULL, TRUE);
break;
case WM_PAINT:
{
PAINTSTRUCT ps;
HDC hdc = BeginPaint(hwnd, &ps);
Gdiplus::Graphics graphics(hdc);
int x = (WIN_WIDTH - cwnd * (RECT_WIDTH + RECT_SPACING)) / 2;
int y = (WIN_HEIGHT - RECT_HEIGHT) / 2;
int i;
for (i = 0; i < cwnd; i++) {
if (i < ssthresh) {
drawRect(graphics, x + i * (RECT_WIDTH + RECT_SPACING), y, RECT_WIDTH, RECT_HEIGHT, SLOW_START_COLOR);
} else {
drawRect(graphics, x + i * (RECT_WIDTH + RECT_SPACING), y, RECT_WIDTH, RECT_HEIGHT, CONGESTION_AVOIDANCE_COLOR);
}
}
if (state == 2) {
drawRect(graphics, x + (cwnd - 1) * (RECT_WIDTH + RECT_SPACING), y, RECT_WIDTH, RECT_HEIGHT, TIMEOUT_COLOR);
}
EndPaint(hwnd, &ps);
}
break;
case WM_DESTROY:
KillTimer(hwnd, ID_TIMER);
PostQuitMessage(0);
break;
default:
return DefWindowProc(hwnd, msg, wParam, lParam);
}
return 0;
}
void drawRect(Gdiplus::Graphics& graphics, int x, int y, int width, int height, COLORREF color)
{
Gdiplus::SolidBrush brush(color);
Gdiplus::Rect rect(x, y, width, height);
graphics.FillRectangle(&brush, rect);
}
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, LPSTR lpCmdLine, int nCmdShow)
{
Gdiplus::GdiplusStartupInput gdiplusStartupInput;
ULONG_PTR gdiplusToken;
Gdiplus::GdiplusStartup(&gdiplusToken, &gdiplusStartupInput, NULL);
WNDCLASS wc;
HWND hwnd;
MSG msg;
// 注册窗口类
ZeroMemory(&wc, sizeof wc);
wc.hbrBackground = (HBRUSH)(COLOR_WINDOW + 1);
wc.hCursor = LoadCursor(NULL, IDC_ARROW);
wc.hInstance = hInstance;
wc.lpfnWndProc = WndProc;
wc.lpszClassName = "MyWindowClass";
wc.style = CS_HREDRAW | CS_VREDRAW;
RegisterClass(&wc);
// 创建窗口
hwnd = CreateWindow("MyWindowClass", "TCP Congestion Control Animation", WS_OVERLAPPEDWINDOW, CW_USEDEFAULT, CW_USEDEFAULT, WIN_WIDTH, WIN_HEIGHT, NULL, NULL, hInstance, NULL);
ShowWindow(hwnd, nCmdShow);
UpdateWindow(hwnd);
// 消息循环
while (GetMessage(&msg, NULL, 0, 0))
{
TranslateMessage(&msg);
DispatchMessage(&msg);
}
Gdiplus::GdiplusShutdown(gdiplusToken);
return (int)msg.wParam;
}
```
运行该程序后,会弹出一个窗口,其中会显示一个拥塞窗口的仿真动画。程序会定时更新拥塞窗口的大小,并根据当前状态绘制不同颜色的矩形来表示不同阶段。可以通过修改程序中的参数来调整拥塞控制算法的参数和动画效果。
相关推荐
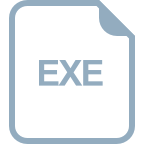














