vue component标签
时间: 2023-08-30 19:10:57 浏览: 82
在 Vue.js 中,可以使用自定义组件来创建可重用的代码块。在模板中使用自定义组件时,需要使用组件标签来引用它们。
例如,如果你创建了一个名为 `my-component` 的组件,可以在模板中使用以下标签来引用它:
```html
<my-component></my-component>
```
你还可以在组件标签中传递 props(属性),例如:
```html
<my-component :prop-name="propValue"></my-component>
```
其中 `prop-name` 是组件的一个 prop 名称,`propValue` 是传递给该 prop 的值。注意,在使用 props 时,需要使用冒号 `:` 来将 prop 名称转换为 JavaScript 表达式。
除了常规的组件标签,Vue.js 还支持自闭合的标签形式,例如:
```html
<my-component />
```
这种自闭合标签的写法与 HTML5 中的自闭合标签相似。但需要注意的是,这种写法只适用于没有子节点的组件。如果组件包含子节点,则必须使用常规的组件标签形式。
相关问题
vue3 component标签传参
在Vue 3中,`<component>` 标签用于动态地加载和渲染组件。如果你想通过这个标签传参给组件,可以利用JavaScript表达式或直接属性绑定的方式来进行。这里有两种常见的方法:
1. **通过props传递**:
当你有一个自定义组件,可以通过`props`属性接收外部传入的数据。例如:
```html
<template>
<div>
<component :is="selectedComponent" :prop1="param1" :prop2="param2"></component>
</div>
</template>
<script setup>
import ChildComponentA from '@/components/ChildComponentA.vue';
import ChildComponentB from '@/components/ChildComponentB.vue';
const selectedComponent = 'ChildComponentA'; // 可以动态切换为'ChildComponentB'
const param1 = 'some value';
const param2 = 'another value';
const components = {
ChildComponentA,
ChildComponentB,
};
</script>
```
2. **使用ref和v-bind动态绑定**:
如果参数值需要在运行时动态设置,可以使用`ref`创建一个引用,然后在`v-bind`中更新其值:
```html
<template>
<div>
<component ref="myComponent" v-bind="{ myProp: myValue }"></component>
</div>
</template>
<script setup>
const myValue = ref('default-value');
// ...
function updateMyParam(newValue) {
myValue.value = newValue;
}
</script>
```
vue中使用component标签传值
在 Vue.js 中,`<component>` 标签是用来动态加载组件的。如果你想通过这个标签向子组件传递数据,你可以使用 `props`(属性)。下面是一个简单的例子:
```html
<template>
<div>
<button @click="loadChildComponent">Load Child Component</button>
<component :is="currentComponent" v-if="currentComponent">
<!-- 通过 props 传递数据 -->
<child-component :message="parentMessage"></child-component>
</v-if>
</div>
</template>
<script>
import ChildComponent from './ChildComponent.vue';
export default {
components: { ChildComponent },
data() {
return {
currentComponent: 'ChildComponent', // 当前显示的组件名
parentMessage: 'Hello from parent!', // 父组件要传递给子组件的数据
};
},
methods: {
loadChildComponent() {
this.currentComponent = 'ChildComponent'; // 动态切换到 ChildComponent 组件
}
}
};
</script>
```
在这个例子中,`:message` 属性告诉子组件 `ChildComponent` 接收名为 `message` 的 prop。当点击按钮加载子组件时,父组件会将 `parentMessage` 数据传递给子组件。
阅读全文
相关推荐
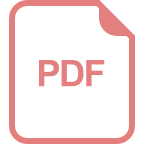
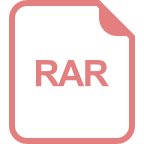













