Java语言实现对文件输入流进行hashmap存储,再将存储内容输入文本文件内,姓氏为key 名字为value
时间: 2024-12-15 17:26:04 浏览: 3
在Java中,你可以使用`HashMap`来存储文件名和对应的姓氏作为键值对,然后使用`FileWriter`和`BufferedWriter`来将这些数据写入到一个新的文本文件中。以下是基本步骤:
1. **读取文件并创建HashMap**:
使用`Scanner`从文件中逐行读取数据,每一行通常包含两个部分(例如,"李四"),将其分割成姓氏和名字,并存储在`HashMap<String, String>`中。
```java
import java.io.*;
import java.util.*;
public class FileHashmap {
public static void main(String[] args) {
HashMap<String, String> map = new HashMap<>();
try (Scanner scanner = new Scanner(new File("input.txt"))) {
while (scanner.hasNextLine()) {
String line = scanner.nextLine();
// 假设每行格式为 "姓+名"
String[] parts = line.split(" ");
map.put(parts[0], parts[1]);
}
} catch (FileNotFoundException e) {
System.err.println("Input file not found");
}
}
}
```
2. **写入HashMap内容到新文件**:
创建一个`FileWriter`对象,如果文件不存在则创建,然后使用`BufferedWriter`将`HashMap`的内容写入。
```java
try (BufferedWriter writer = new BufferedWriter(new FileWriter("output.txt"))) {
for (Map.Entry<String, String> entry : map.entrySet()) {
writer.write(entry.getKey() + "\t" + entry.getValue());
writer.newLine(); // 添加换行符
}
} catch (IOException e) {
System.err.println("Error writing to output file");
}
```
在这个例子中,假设你的输入文件`input.txt`的格式是这样的:
```
李四
张三
王五
```
运行上述程序后,输出文件`output.txt`将会包含:
```
李 四
张 三
王 五
```
阅读全文
相关推荐
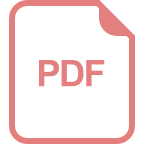
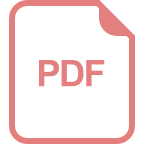
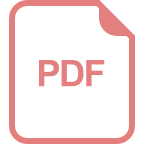
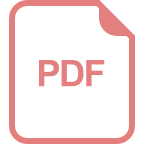
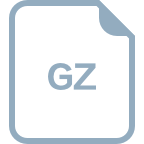
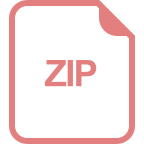
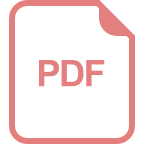
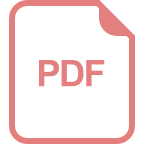











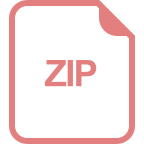